NYU CS-UY 1134 Lab1
Vitamins (10 minutes)
Write the output for the following lines of code given the Student class. (10 minutes).
为以下代码写输出,给定的是
Student
类。(10分钟)。
class Student:
def __init__(self, name="student", age=18):
self.name = name
self.age = age
self.courses = []
def add_course(self, course):
self.courses.append(course)
def remove_course(self, course):
if course in self.courses:
self.courses.remove(course)
print("Removed Course:", course)
else:
print("Course Not Found:", course)
def __repr__(self): # str representation needed for print( )
info = "Name: " + self.name
info += "\nAge: " + str(self.age)
info += "\nCourses: " + " , ".join(self.courses)
return info + "\n"
# 定义一个名为Student的类
class Student:
# 初始化方法,用于构造对象时的初始化操作
# 参数:name默认值为'student',age默认值为18
def __init__(self, name="student", age=18): # 将参数 name 的值赋值给对象的 name 属性 == self.name >>> 为了实现类当中,函数参数的互相调用
# 将参数name的值赋给对象的name属性
self.name = name
# 将参数age的值赋给对象的age属性
self.age = age
# 初始化courses属性为一个空列表,表示学生所选的课程
self.courses = []
# 方法,用于添加课程到学生的courses列表
def add_course(self, course):
# 将course参数的值添加到courses列表中
self.courses.append(course)
# 方法,用于从学生的courses列表中移除课程
def remove_course(self, course):
# 如果course在courses列表中
if course in self.courses:
# 从courses列表中移除course
self.courses.remove(course)
# 打印移除的课程信息
print("Removed Course:", course)
# 如果course不在courses列表中
else:
# 打印课程未找到的信息
print("Course Not Found:", course)
# 重载repr方法,当我们尝试print一个Student对象时,会返回这个方法的返回值
def __repr__(self):
# 将学生的name属性连接到字符串"Name: "后面
info = "Name: " + self.name
# 将学生的age属性(需要先转为字符串)连接到字符串"Age: "后面
info += "\nAge: " + str(self.age)
# 将学生的courses列表转为字符串(用逗号和空格连接)并连接到字符串"Courses: "后面
info += "\nCourses: " + " , ".join(self.courses)
# 返回完整的字符串,末尾添加一个换行符
return info + "\n"
# 创建一个Student对象,age设置为16,name使用默认值'student'
peter = Student(16)
# 打印peter对象的name和age属性
print(peter.name, peter.age)
# 创建一个Student对象,name设置为"Peter Parker",age使用默认值18
peter = Student("Peter Parker")
# 打印peter对象的name和age属性
print(peter.name, peter.age)
peter = Student(16)
print(peter.name, peter.age)
# out
16 18
peter = Student("Peter Parker")
print(peter.name, peter.age)
# out
Peter Parker 18
peter = Student(age=16)
print(peter.name, peter.age)
# out
student 16
peter.name = "Peter Parker"
print(peter)
# out
Name: Peter Parker
Age: 16
Courses:
peter.add_course("Algebra")
peter.add_course("Chemistry")
print(peter)
# out
Name: Peter Parker
Age: 16
Courses: Algebra , Chemistry
peter.add_course("Physics")
peter.remove_course("Spanis")
# out
Course Not Found: Spanis
tom = Student("Tom holland")
tom.courses = peter.courses
tom.add_course("Economics")
peter.remove_course("Chemistry")
print(peter.courses)
print(tom.courses)
peter.name, tom.name = tom.name, peter.name
print(peter.name, peter.age)
print(tom.name, tom.age)
Coding
In this section, it is strongly recommended that you solve the problem on paper before writing code. This will be good practice for when you write code by hand on the exams.
- Implement the following function (30 minutes):
def can_construct(word, letters): """
word - type: str
letters - type: str
return value - type: bool
"""
This function is passed in a string containing a word, and another string containing letters in your hand. When called, it will return True if the word can be constructed with the letters provided; otherwise, it will return False.
Notes:
- Each letter provided can only be used one.
- You may assume that the word and letters will only contain lower-case letters.
- You may not use a dictionary for this question.
- Hint : Try to think about how you can use a list to implement a dictionary
ex) can_construct("apples", "aples") will return False.
ex) can_construct("apples", "aplespl") will return True.
详情
def can_construct(word, letters):
"""
word - 类型: str
letters - 类型: str
返回值 - 类型: bool
"""
这个函数会传入一个包含单词的字符串和另一个包含你手里的字母的字符串。当被调用时,如果可以用所提供的字母构建该单词,它将返回True;否则,它将返回False。
注意:
- 每个提供的字母只能使用一次。
- 您可以假设 word 和 letters 只包含小写字母。
- 对于这个问题,您不能使用字典。
- 提示: 尝试思考如何使用列表来实现字典
例如 can_construct("apples", "aples") 将返回False。
例如 can_construct("apples", "aplespl") 将返回True。
def can_construct(word, letters):
# 将letters的每个字符转化为列表
letters_list = list(letters)
# 对于word中的每个字符,检查它是否在letters_list中
for char in word:
if char in letters_list:
# 如果字符在letters_list中,从中移除该字符
letters_list.remove(char)
else:
# 如果字符不在letters_list中,返回False
return False
# 如果所有字符都在letters_list中,返回True
return True
公众号:AI悦创【二维码】
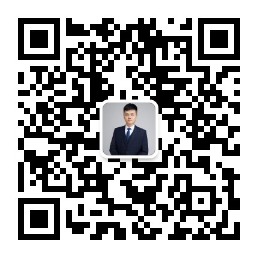
AI悦创·编程一对一
AI悦创·推出辅导班啦,包括「Python 语言辅导班、C++ 辅导班、java 辅导班、算法/数据结构辅导班、少儿编程、pygame 游戏开发、Web、Linux」,全部都是一对一教学:一对一辅导 + 一对一答疑 + 布置作业 + 项目实践等。当然,还有线下线上摄影课程、Photoshop、Premiere 一对一教学、QQ、微信在线,随时响应!微信:Jiabcdefh
C++ 信息奥赛题解,长期更新!长期招收一对一中小学信息奥赛集训,莆田、厦门地区有机会线下上门,其他地区线上。微信:Jiabcdefh
方法一:QQ
方法二:微信:Jiabcdefh
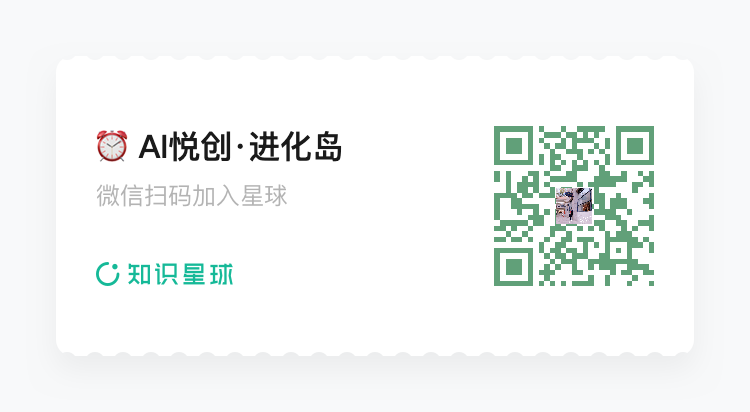
- 0
- 0
- 0
- 0
- 0
- 0