CUH403CMD OO Programming
Review this week’s lecture material and complete the following tasks:
TASK 1
Consider the snippet of code below (this is related to the questions 1-3 below):
public class Person
{
public void Walk()
{
Console.WriteLine("This is a Person walking");
}
}
public class Student : Person
{
public void Walk()
{
Console.WriteLine("This is a Student walking");
}
}
public class Teacher : Person
{
public void Walk()
{
Console.WriteLine("This is a Teacher walking");
}
}
ANSWER the following questions (try to answer BEFORE coding in MS. VS):
- Which of the following declarations will cause a compile-time error:
a. Person instanceA = new Person();
b. Person instanceB = new Teacher();
c. Theacher instanceC = new Teacher();
d. Teacher instanceD = new Person();
e. Student instanceE = new Teacher();
- Consider the following declarations:
a. Person John = new Person();
b. Teacher Thomas = new Teacher();
c. Person Luisa = new Teacher();
Identify the following assignments as valid or invalid:
a. John = Thomas;
b. Thomas = (Teacher)Luisa;
c. John = (Teacher)Thomas;
- Consider the following declarations:
a. Person John = new Person();
b. Teacher Thomas = new Teacher();
c. Person Luisa = new Teacher();
Predict the output of the following code lines:
a. John = Thomas;
b. John.Walk();
c. Luisa.Walk();
d. Thomas.Run();
CODE a simple program to demonstrate that your answers 1 to 3 are correct. You should provide a test class to demonstrate the functionality.
详情
Q1
a.
Person instanceA = new Person();
这个声明不会引起编译时错误。它创建了一个Person
类型的实例。b.
Person instanceB = new Teacher();
这个声明不会引起编译时错误。Teacher
是Person
的子类,所以可以将一个Teacher
实例赋值给一个Person
类型的变量。c.
Teacher instanceC = new Teacher();
这个声明的拼写错误,应该是Teacher
而不是Theacher
,如果更正为Teacher
,则不会引起编译时错误。它创建了一个Teacher
类型的实例。d.
Teacher instanceD = new Person();
这个声明会引起编译时错误。不能将父类的实例直接赋值给子类的变量,除非使用显式类型转换。e.
Student instanceE = new Teacher();
这个声明会引起编译时错误。Student
和Teacher
都继承自Person
,但它们之间没有直接的继承关系,不能将一个类的实例赋值给另一个类的实例,除非它们有直接的继承关系或者通过某种方式可以兼容(例如接口实现)。
Q2
a.
John = Thomas;
有效。Thomas
是Teacher
的实例,而Teacher
继承自Person
。所以,一个Teacher
实例可以赋值给Person
类型的变量。b.
Thomas = (Teacher)Luisa;
有效。尽管Luisa
被声明为Person
类型,但它实际上是一个Teacher
的实例(new Teacher();
)。通过显式类型转换,可以将Luisa
赋值给Teacher
类型的变量。c.
John = (Teacher)Thomas;
无效。这里尝试将Thomas
(一个Teacher
实例)转换为Teacher
类型然后赋值给一个Person
类型的变量。尽管转换本身是多余的(因为Thomas
已经是Teacher
类型),但它不会引起编译错误。问题的描述有误,实际上这种赋值是可以进行的,因为Teacher
继承自Person
。
Q3
a. John = Thomas;
- 这行代码本身不产生输出,它只是赋值操作。
b. John.Walk();
- 输出This is a Teacher walking
。因为John
引用的是Thomas
对象(一个Teacher
实例)。
c. Luisa.Walk();
- 输出This is a Teacher walking
。因为Luisa
虽然被声明为Person
类型,但实际上是Teacher
的一个实例。
d. Thomas.Run();
- 这行代码会导致编译错误,因为在Teacher
类中没有定义Run
方法。
综上所述:
- 将导致编译时错误的声明是 d 和 e。
- 赋值操作a和b是有效的;c 在语义上是多余的,但不会引起错误。
- 预测的输出是:
- b.
This is a Teacher walking
- c.
This is a Teacher walking
- d. 编译错误,因为
Run
方法不存在。
- b.
TASK 2
You are developing code for a method that calculates the discount for the items sold. You name the method CalculateDiscount. The method defines a variable, percentValue of the type double. You need to make sure that percentValue is accessible only within the CalculateDiscount method. Which access modifier should you use when defining the percentValue variable?
a. private
b. protected
c. internal
d. public
CODE a simple program to demonstrate that your answers. You should provide a test class to demonstrate the functionality
Make notes for your own reference.
// // 引入System命名空间,它包含基础类和基本功能
// using System;
// // 定义一个名为DiscountCalculator的公共类,用于计算商品折扣
// public class DiscountCalculator
// {
// // 定义一个公共方法CalculateDiscount,它接收两个double类型的参数:
// // originalPrice代表商品的原价,discountPercent代表折扣百分比
// public double CalculateDiscount(double originalPrice, double discountPercent)
// {
// // 在方法内部定义一个局部变量percentValue,将折扣百分比转换为小数形式
// // 由于是方法内的局部变量,它只能在这个方法内被访问和使用
// double percentValue = discountPercent / 100;
// // 计算折扣金额,即原价乘以折扣率
// double discountAmount = originalPrice * percentValue;
// // 计算并返回打折后的价格,即原价减去折扣金额
// return originalPrice - discountAmount;
// }
// }
// // 定义一个测试类TestClass,包含主程序入口点Main方法
// public class TestClass
// {
// // Main方法是程序的入口点,此处用static void Main(string[] args)定义
// public static void Main(string[] args)
// {
// // 创建DiscountCalculator类的实例,以便调用其中的方法
// DiscountCalculator calculator = new DiscountCalculator();
// // 设置一个示例原价100元,折扣为20%
// double originalPrice = 100;
// double discountPercent = 20;
// // 调用CalculateDiscount方法计算打折后的价格
// // 将计算结果赋值给finalPrice变量
// double finalPrice = calculator.CalculateDiscount(originalPrice, discountPercent);
// // 使用Console.WriteLine输出计算结果到控制台
// // 输出的字符串包括原价、折扣百分比和打折后的价格
// Console.WriteLine($"原价为{originalPrice}元,折扣为{discountPercent}%,打折后的价格为{finalPrice}元。");
// }
// }
using System;
public class DiscountCalculator
{
// 定义一个私有变量,虽然这不是题目要求的局部变量,但展示了如何使用访问修饰符
private double percentValue;
// 计算折扣的方法,现在通过设置percentValue值来使用
public double CalculateDiscount(double originalPrice, double discountPercent)
{
this.percentValue = discountPercent / 100;
double discountAmount = originalPrice * this.percentValue;
return originalPrice - discountAmount;
}
}
public class TestClass
{
public static void Main(string[] args)
{
DiscountCalculator calculator = new DiscountCalculator();
// 假设原价为100元,折扣为20%
double originalPrice = 100;
double discountPercent = 20;
double finalPrice = calculator.CalculateDiscount(originalPrice, discountPercent);
Console.WriteLine($"原价为{originalPrice}元,折扣为{discountPercent}%,打折后的价格为{finalPrice}元。");
}
}
TASK 1. CONSIDER the coding exercise:
- launch Microsoft Visual Studio and create a new Visual C# Console Application Project, then perform the following steps:
- Add a new Visual C# class named Rectangle to the project
- Replace the code for the Rectangle class with the following code:
class Rectangle {
private double length;
private double width;
public Rectangle(double l, double w) {
length = l;
width = w;
}
public double GetArea() {
return length * width;
}
}
Next step:
Use the console application project, which you have just created and then perform the following steps:
- Modify the code of the Program class to the following:
class Program {
static void Main(string[] args) {
Rectangle rect = new Rectangle(10.0, 20.0);
double area = rect.GetArea();
Console.WriteLine($"Area of Rectangle: {area}");
}
}
As previously – test your application. Select Debug > Start Without Debugging. A console window displays the calculated area of the rectangle. When you select Debug > Start Debugging (or Ctrl+F5), the console window will close as soon as the program ends and you might not get a chance to review the output.
SAVE your project (or Ctrl + S).
公众号:AI悦创【二维码】
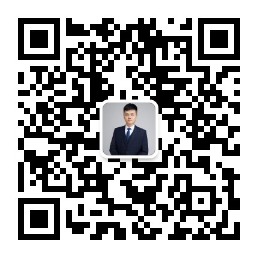
C:::
AI悦创·编程一对一
AI悦创·推出辅导班啦,包括「Python 语言辅导班、C++ 辅导班、java 辅导班、算法/数据结构辅导班、少儿编程、pygame 游戏开发、Web、Linux」,全部都是一对一教学:一对一辅导 + 一对一答疑 + 布置作业 + 项目实践等。当然,还有线下线上摄影课程、Photoshop、Premiere 一对一教学、QQ、微信在线,随时响应!微信:Jiabcdefh
C++ 信息奥赛题解,长期更新!长期招收一对一中小学信息奥赛集训,莆田、厦门地区有机会线下上门,其他地区线上。微信:Jiabcdefh
方法一:QQ
方法二:微信:Jiabcdefh
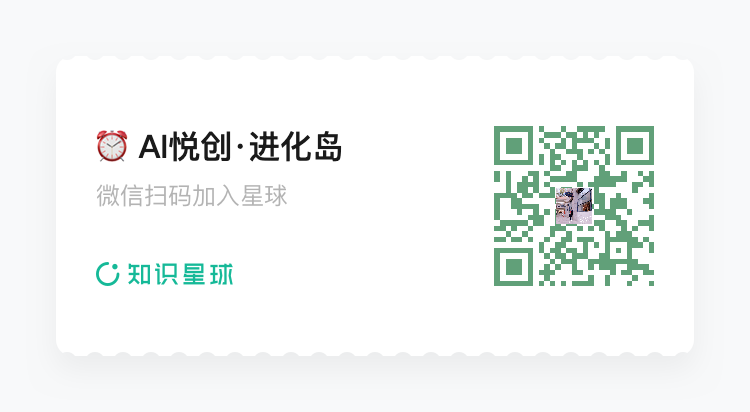
- 0
- 0
- 0
- 0
- 0
- 0