02-Control statements
Exercise 02.1 (if-else)
Consider the following assessment criteria which map a score out of 100 to an
assessment grade:
Grade | Raw score (/100) |
---|---|
Excellent | |
Very good | and |
Good | and |
Need improvement | and |
Did you try? |
Write a program that, given an a score, prints the appropriate grade. Print an error message if the input score is greater than 100 or less than zero.
# Score from user
score = 72
...
考虑以下的评分标准,它将一个满分为100的分数映射到一个评估等级:
等级 | 原始分数 (/100) |
---|---|
优秀 | |
非常好 | 且 |
良好 | 且 |
需要改进 | 且 |
你有尝试吗? |
编写一个程序,给定一个分数,输出相应的等级。如果输入的分数大于100或小于0,打印一个错误信息。
score = float(input("请输入你的分数(0到100之间):"))
if score > 100 or score < 0:
print("错误:分数应在0到100之间")
elif score >= 85:
print("等级:优秀")
elif score >= 76.5:
print("等级:非常好")
elif score >= 64:
print("等级:良好")
elif score >= 40:
print("等级:需要改进")
else:
print("等级:你有尝试吗?")
score = float(input("Enter your score (between 0 to 100): "))
if score > 100 or score < 0:
print("Error: The score should be between 0 and 100.")
elif score >= 85:
print("Grade: Excellent")
elif score >= 76.5:
print("Grade: Very good")
elif score >= 64:
print("Grade: Good")
elif score >= 40:
print("Grade: Need improvement")
else:
print("Grade: Did you try?")
Exercise 02.2 (bisection)
Bisection is an iterative method for finding approximate roots of a function. Say we know that the function has one root between and (). We then:
Evaluate at the midpoint , i.e. compute
Evaluate
if :
must change sign somewhere between and , hence the root must lie between
and , so set .else:
must change sign somewhere between and , so set
.
The above steps can be repeated a specified number of times, or until
is below a tolerance, with being the approximate root.
Task
The function
has one root in the range .
- Use the bisection method to find an approximate root using 20 iterations
(use afor
loop). - Use the bisection method to find an approximate root such that
and report the number of iterations
required (use awhile
loop).
Store the approximate root using the variable x_mid
, and store using the variable f
.
Hint: Use abs
to compute the absolute value of a number, e.g. y = abs(x)
assigns the absolute value of x
to y
.
二分法是一种迭代方法,用于查找函数的近似根。假设我们知道函数 在 和 之间有一个根 ()。我们可以:
在中点 处评估 ,即计算
计算
如果 :
必须在 和 之间某处改变符号,因此根必须位于 和 之间,所以设置 。
否则:
必须在 和 之间某处改变符号,所以设置 。
以上步骤可以重复指定的次数,或者直到 低于某个容忍度,此时 为近似根。
任务
函数
在范围 内有一个根。
- 使用二分法在 20 次迭代中找到一个近似根 (使用
for
循环)。 - 使用二分法找到一个近似根 ,使得 ,并报告所需的迭代次数(使用
while
循环)。
使用变量 x_mid
存储近似根,并使用变量 f
存储 。
提示: 使用 abs
计算数字的绝对值,例如 y = abs(x)
将 x
的绝对值赋给 y
。
# 初始端点值
x0 = 0.0 # 初始的x0值
x1 = 2.0 # 初始的x1值
# 进行20次迭代
for n in range(20):
# 计算中点值
x_mid = (x0 + x1) / 2 # 根据二分法计算中间值
# 计算在(i)左端点以及(ii)中点处的函数值
# 注意:在这里我们使用了函数f(x)的定义来计算f0和f
f0 = (-x0**5) / 10 + x0**3 - 10 * x0**2 + 4 * x0 + 7 # 在x0处的函数值
f = (-x_mid**5) / 10 + x_mid**3 - 10 * x_mid**2 + 4 * x_mid + 7 # 在中点x_mid处的函数值
# 根据f0和f的乘积来确定新的区间界限
# 1. 如果f0和f的乘积小于0,那么根必定存在于[x0, x_mid]之间,所以我们更新x1为x_mid
# 2. 如果f0和f的乘积大于或等于0,那么根必定存在于[x_mid, x1]之间,所以我们更新x0为x_mid
if f0 * f < 0:
x1 = x_mid
else:
x0 = x_mid
# 输出当前迭代的结果
print(n, x_mid, f)
# Initial end points
x0 = 0.0
x1 = 2.0
tol = 1.0e-6
error = tol + 1.0
# Iterate until tolerance is met
counter = 0
while error > tol:
# 计算中点
x_mid = (x0 + x1) / 2
# 在(i)左端点和(ii)中点处评估函数
f0 = (x0**5) / 10 + x0**3 - 10 * x0**2 + 4 * x0 + 7
f = (x_mid**5) / 10 + x_mid**3 - 10 * x_mid**2 + 4 * x_mid + 7
# 更新error
error = abs(f)
# 判断根的位置
if f0 * f < 0:
x1 = x_mid
else:
x0 = x_mid
counter += 1
# Guard against an infinite loop
if counter > 1000:
print("Oops, iteration count is very large. Breaking out of while loop.")
break
print(counter, x_mid, error)

Exercise 02.3 (series expansion)
For the series:
converges.
Using a
for
statement, approximate using 30 terms in the series expansion and report the absolute error.Using a
while
statement, compute how many terms in the series are required to approximate to within .
Store the absolute value of the error in the variable error
.
Hints
To compute the factorial, use the Python math
module:
import math
nfact = math.factorial(10)
You only need import math
once at the top of your program. Standard modules, like math
, will be explained in a later
# Import the math module to access math.factorial
import math
# Value of x (such that (1 - x) = 0.16
x = -0.84
# Initialise approximation of the function
approx_f = 0.0
...
print("The error is:")
print(error)
## test ##
assert error < 1.0e-2
# Import the math module to access math.sin and math.factorial
import math
# Value of x (such that (1 - x) = 0.16)
x = -0.84
# Tolerance and initial error (this just needs to be larger than tol)
tol = 1.0e-5
error = tol + 1.0
# Initialise approximation of function
approx_f = 0.0
# Initialise counter
n = 0
# Loop until error satisfies tolerance, with a check to avoid
# an infinite loop
while error > tol and n < 1000:
...
# Increment counter
n += 1
print("\nThe error is:", error)
print("Number of terms in series:", n)
## test ##
assert error <= 1.0e-5
对于 的序列:
该级数收敛。
使用
for
语句,用该级数展开的前30项近似计算 ,并报告绝对误差。使用
while
语句,计算需要多少级数的项才能将 近似到 内。
将误差的绝对值存储在变量 error
中。
提示
要计算阶乘,请使用Python的 math
模块:
import math
nfact = math.factorial(10)
你只需要在程序的开头使用一次 import math
。标准模块,例如 math
,将在后面进行解释。
我们可以使用上述伪代码来实现对给定函数的数列展开。首先,我们用 for
循环来近似计算 的值,然后用 while
循环来找出满足误差小于 的数列项数。
(1) 使用 for
循环:
# 导入math模块以使用math.factorial
import math
# x的值 (这样 (1 + x) = 1.84)
x = 0.84
# 初始化函数的近似值
approx_f = 0.0
# 使用30个数列项
for n in range(30):
term = ((-1)**n * math.factorial(2*n)) / (4**n * (math.factorial(n))**2) * x**n
approx_f += term
# 计算真实值和误差
true_value = 1 / (0.16)**0.5
error = abs(true_value - approx_f)
print("使用30个数列项的近似值为:", approx_f)
print("真实值为:", true_value)
print("误差为:", error)
## 测试 ##
assert error < 1.0e-2
(2) 使用 while
循环:
# x的值 (这样 (1 + x) = 1.84)
x = 0.84
# 容差和初始误差 (只需要确保比容差大)
tol = 1.0e-5
error = tol + 1.0
# 初始化函数的近似值
approx_f = 0.0
# 初始化计数器
n = 0
# 循环直到误差满足容差,同时检查以避免无限循环
while error > tol and n < 1000:
term = ((-1)**n * math.factorial(2*n)) / (4**n * (math.factorial(n))**2) * x**n
approx_f += term
error = abs(true_value - approx_f)
# 增加计数器
n += 1
print("\n误差为:", error)
print("数列项数:", n)
## 测试 ##
assert error <= 1.0e-5
公众号:AI悦创【二维码】
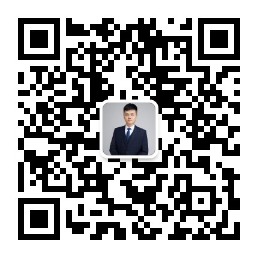
AI悦创·编程一对一
AI悦创·推出辅导班啦,包括「Python 语言辅导班、C++ 辅导班、java 辅导班、算法/数据结构辅导班、少儿编程、pygame 游戏开发、Web、Linux」,全部都是一对一教学:一对一辅导 + 一对一答疑 + 布置作业 + 项目实践等。当然,还有线下线上摄影课程、Photoshop、Premiere 一对一教学、QQ、微信在线,随时响应!微信:Jiabcdefh
C++ 信息奥赛题解,长期更新!长期招收一对一中小学信息奥赛集训,莆田、厦门地区有机会线下上门,其他地区线上。微信:Jiabcdefh
方法一:QQ
方法二:微信:Jiabcdefh
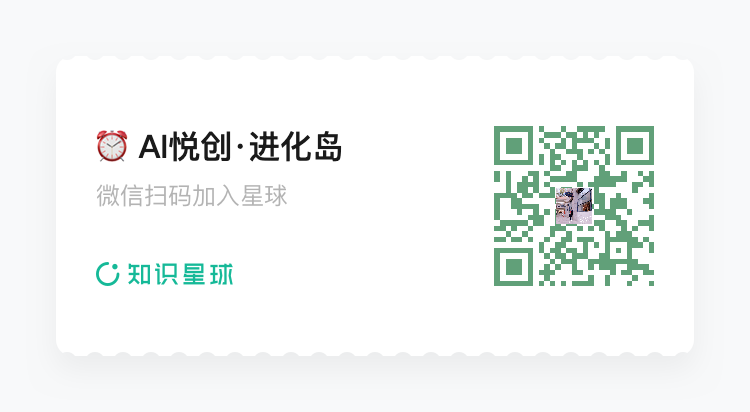
- 0
- 0
- 0
- 0
- 0
- 0