Problem 1
Q1
In this exercise, you are given the following tuple:
my_tuple = (0, 1, 2, "hi", 4, 5)
Make it so that it instead holds:
(0, 1, 2, 3, 4, 5)
Remember that you cannot change a tuple, so this will not work:
# Error!
my_tuple[3] = 3
You also shouldn’t just do something like this:
# Incorrect! Too easy!
my_tuple = (0, 1, 2, 3, 4, 5)
Instead, use slices of the old tuple, and concatenate them with the element you want to add. Note that you should not just add the integer 3
, but rather the tuple (3,)
.
在这个练习中,给你以下元组:
my_tuple = (0, 1, 2, "hi", 4, 5)
请将其修改为:
(0, 1, 2, 3, 4, 5)
记住,你不能修改一个元组,所以这样是不行的:
# 错误!
my_tuple[3] = 3
你也不应该只是这样做:
# 错误的!太简单了!
my_tuple = (0, 1, 2, 3, 4, 5)
相反,使用旧元组的切片,并与你想要添加的元素连接起来。注意,你不应该只添加整数3
,而应该添加元组(3,)
。
my_tuple = (0, 1, 2, "hi", 4, 5)
new_tuple = my_tuple[:3] + (3,) + my_tuple[4:]
print(new_tuple)
Q2
Help your friend write their bibliography!
Write the function called citation
that takes a tuple like this as input:
("Martin", "Luther", "King, Jr.")
and returns a name with the format:
Last, First Middle
In this example, the output should be:
King, Jr., Martin Luther
Here are some more examples:
citation(("J.", "D.", "Salinger"))
# => "Salinger, J. D."
citation(("Ursula", "K.", "Le Guin"))
# => "Le Guin, Ursula K."
citation(("J.", "K.", "Rowling"))
# => "Rowling, J. K."
# fill in the `citation` function to return the author's name in the correct format
def citation(author_name):
return ""
编写一个名为 citation
的函数,它接受如下格式的元组作为输入:
("Martin", "Luther", "King, Jr.")
并返回这样格式的名字:
姓, 名 中间名
在此示例中,输出应为:
King, Jr., Martin Luther
这里有一些更多的示例:
citation(("J.", "D.", "Salinger"))
# => "Salinger, J. D."
citation(("Ursula", "K.", "Le Guin"))
# => "Le Guin, Ursula K."
citation(("J.", "K.", "Rowling"))
# => "Rowling, J. K."
# 填写 `citation` 函数以返回正确格式的作者姓名
def citation(author_name):
return ""
我们可以通过元组的索引来取出姓、名和中间名,并按照要求的格式将它们重新组合成一个字符串。
下面是完成这一任务的函数:
def citation(name_tuple):
# 从元组中取出名、中间名和姓
first_name = name_tuple[0]
middle_name = name_tuple[1]
last_name = name_tuple[2]
# 使用字符串的格式化方法,按照"姓, 名 中间名"的格式组合它们
formatted_name = "{}, {} {}".format(last_name, first_name, middle_name)
return formatted_name
# 测试函数
print(citation(("Martin", "Luther", "King, Jr."))) # 输出:King, Jr., Martin Luther
print(citation(("J.", "D.", "Salinger"))) # 输出:Salinger, J. D.
print(citation(("Ursula", "K.", "Le Guin"))) # 输出:Le Guin, Ursula K.
print(citation(("J.", "K.", "Rowling"))) # 输出:Rowling, J. K.
该函数首先从输入的元组中提取名、中间名和姓,然后使用字符串的格式化方法来组合它们,从而得到所需的格式。
# fill in the `citation` function to return the author's name in the correct format
def citation(author_name):
first_name = author_name[0]
middle_name = author_name[1]
last_name = author_name[2]
# user_name = ""first_name + ", "+ last_name + middle_name""
user_name = last_name + ", " + first_name + " " + middle_name
return user_name
r = citation(("J.", "D.", "Salinger"))
print(r)
Q3
Create a list of your favorite 4 movies. Print out the 0th element in the list. Now set the 0th element to be “Star Wars” and try printing it out again.
创建你最喜欢的4部电影的列表。打印列表中的第0个元素。现在将第0个元素设置为“星球大战”,然后再试着打印它。
# 创建一个包含4部电影的列表
favorite_movies = ["Movie1", "Movie2", "Movie3", "Movie4"]
# 打印列表中的第0个元素
print(favorite_movies[0])
# 将第0个元素设置为“星球大战”
favorite_movies[0] = "星球大战"
# 再次打印列表中的第0个元素
print(favorite_movies[0])
Q4
What does the following code print?
time_of_day = ["morning", "afternoon", "evening"]
for word in time_of_day:
print("Good " + word)
Q5
Write the function max_int_in_list
that takes a list of ints and returns the biggest int in the list. You can assume that the list has at least one int in it. A call to this function would look like:
my_list = [5, 2, -5, 10, 23, -21]
biggest_int = max_int_in_list(my_list)
# biggest_int is now 23
Do not use the built-in function max
in your program!
Hint: The highest number in the list might be negative! Make sure your function correctly handles that case.
def max_int_in_list(lst):
# 从列表的第一个元素开始
biggest = lst[0]
# 遍历列表中的每一个元素
for num in lst:
if num > biggest:
biggest = num
return biggest
my_list = [5, 2, -5, 10, 23, -21]
biggest_int = max_int_in_list(my_list)
print(biggest_int) # 输出应该是23
Q6
Your friend really likes talking about owls. Write a function owl_count
that takes a block of text and counts how many words they say have word “owl” in them. Any word with “owl” in it should count, so “owls,” “owlette,” and “howl” should all count.
Here’s what an example run of your program might look like:
text = "I really like owls. Did you know that an owl's eyes are more than twice as big as the eyes of other birds of comparable weight? And that when an owl partially closes its eyes during the day, it is just blocking out light? Sometimes I wish I could be an owl."
owl_count(text)
# => 4
Hints
You will need to use the split
method!
def owl_count(text):
# 将文本转换为小写并分割成单词
words = text.lower().split()
# 使用列表推导计算包含“owl”的单词数
owl_words = [word for word in words if 'owl' in word]
return len(owl_words)
text = "I really like owls. Did you know that an owl's eyes are more than twice as big as the eyes of other birds of comparable weight? And that when an owl partially closes its eyes during the day, it is just blocking out light? Sometimes I wish I could be an owl."
print(owl_count(text)) # 输出应该是4
Q7
Words are way more edgy when you replace the letter i with an exclamation point!
Write the function exclamations
that takes a string and then returns the same string with every lowercase i replaced with an exclamation point. Your function should:
- Convert the initial string to a list
- Use a
for
loop to go through your list element by element- Whenever you see a lowercase i, replace it with an exclamation point in the list
- Return the stringified version of the list when your
for
loop is finished
Here’s what an example run of your program might look like:
exclamations("I like music.")
# => I l!ke mus!c.
exclamations("Mississippi")
# => M!ss!ss!pp!
def exclamations(s):
# 将字符串转换为列表
lst = list(s)
# 使用for循环逐个检查列表中的元素
for i in range(len(lst)):
if lst[i] == 'i':
lst[i] = '!'
# 使用join方法将列表转回为字符串
return ''.join(lst)
# 示例
print(exclamations("I like music.")) # 输出应该是:I l!ke mus!c.
print(exclamations("Mississippi")) # 输出应该是:M!ss!ss!pp!
Q8
Your friend wants to try to make a word ladder! This is a list of words where each word has a one-letter difference from the word before it. Here’s an example:
cat
cot
cog
log
Write a program to help your friend. It should do the following:
- Ask your friend for an initial word
- Repeatedly ask them for an index and a letter
- You should replace the letter at the index they provided with the letter they enter
- You should then print the new word
- Stop asking for input when the user enters -1 for the index
Here’s what should be happening behind the scenes:
- You should have a function,
get_index
, that repeatedly asks the user for an index until they enter a valid integer that is within the acceptable range of indices for the initial string. (If they enter a number out of range, you should replyinvalid index
.) - You should have another function,
get_letter
, that repeatedly asks the user for a letter until they enter exactly one lowercase letter. (If they enter more than one character, you should replyMust be exactly one character!
. If they enter a capital letter, you should replyCharacter must be a lowercase letter!
.) - You should store a list version of the current word in a variable. This is what you should update each time the user swaps out a new letter.
- Each time you have to print the current word, print the string version of the list you are keeping in your variable.
Here’s what an example run of your program might look like:
Enter a word: cat
Enter an index (-1 to quit): 1
Enter a letter: o
cot
Enter an index (-1 to quit): 2
Enter a letter: g
cog
Enter an index (-1 to quit): 5
Invalid index
Enter an index (-1 to quit): -3
Invalid index
Enter an index (-1 to quit): 0
Enter a letter: L
Character must be a lowercase letter!
Enter a letter: l
log
Enter an index (-1 to quit): -1
Q9
In the Librarian exercise, you asked the user for five last names, and you then printed a list of those names in sorted order.
In this exercise, you will ask the user for five full names. You should still print a list of last names in sorted order.
Each time you retrieve a name from the user, you should use the split method and the right index to extract just the last name. You can then add the last name to the list of last names that you will ultimately sort and print.
Here’s what an example run of your program might look like:
Name: Maya Angelou
Name: Chimamanda Ngozi Adichie
Name: Tobias Wolff
Name: Sherman Alexie
Name: Jane Austen
['Adichie', 'Alexie', 'Angelou', 'Austen', 'Wolff']
# 初始化一个空列表来存放姓氏
last_names = []
# 循环5次,每次获取用户输入的完整名字
for _ in range(5):
full_name = input("Name: ")
# 使用split方法分割名字,获取最后一个元素作为姓氏
last_name = full_name.split()[-1]
# 添加姓氏到列表中
last_names.append(last_name)
# 对姓氏列表进行排序
last_names.sort()
# 打印排序后的姓氏列表
print(last_names)
Q10
This program is an extension of your previous ‘Owls’ program.
In addition to just reporting how many words contained the word owl, you should report the indices at which the words occurred!
Here’s what an example run of your program might look like:
Enter some text: owls are so cool! I think snowy owls might be my favorite. Or maybe spotted owls.
There were 3 words that contained "owl".
They occurred at indices: [0, 7, 15]
As you can see from the output, you’ll have to use another list to store the indices where you found the words containing “owl”.
The enumerate
function might also come in handy!
# 获取用户输入的文本
text = input("Enter some text: ")
# 使用split方法分割文本
words = text.split()
# 初始化空列表来存放索引
indices = []
# 使用enumerate函数遍历单词及其索引
for index, word in enumerate(words):
# 如果单词中包含"owl",则把索引添加到列表中
if 'owl' in word:
indices.append(index)
# 输出结果
print(f"There were {len(indices)} words that contained \"owl\".")
print(f"They occurred at indices: {indices}")
Q11
Write a program that simulates a coin flipping. Each time the program runs, it should print either “Heads” or “Tails”.
There should be a 0.5 probability that “Heads” is printed, and a 0.5 probability that “Tails” is printed.
There is no actual coin being flipped inside of the computer, and there is no simulation of a metal coin actually flipping through space. Instead, we are using a simplified model of the situation, we simply generate a random probability, with a 50% chance of being true, and a 50% chance of being false.
import random
def coin_flip():
if random.random() < 0.5:
return "Heads"
else:
return "Tails"
print(coin_flip())
Math
7.4.16 AP Practice: List Procedures
Question: 1
The AP Exam uses the following format to insert an item to a list and to generate a random integer from a to b, including a and b.
INSERT(aList, i, value)
RANDOM(a, b)
Given the following code segment, how can you best describe its behavior?
i ← 1
FOR EACH x IN list
{
REMOVE(list, i)
random ← RANDOM(1, LENGTH(list))
INSERT(list, random, x)
i ← i + 1
}
- This code shuffles the order of the numbers in the list by removing them and inserting them back in a random place.
- This code removes all of the numbers in the list and inserts random numbers in random places in the list.
- This code errors by trying to access an element at an index greater than the length of the list.✅
Question: 2
The AP Exam uses the following format to iterate through a list.
FOR EACH item IN aList
{
<block of statements>
}
Given the following code segment, how can you best describe its behavior?
result ← 0
FOR EACH n IN list
{
IF (n MOD 2 = 0)
{
result ← result + n
}
}
DISPLAY result
A. This code displays the count of even numbers in list
B. This code displays the sum of the even numbers in list
✅
C. This code displays the sum of the numbers at the even indices of list
D. This code results in an error
Question: 3
The AP Exam uses the following format to add an item to list and to find the length of a list.
APPEND(aList, value)
LENGTH(aList)
Given the following code segment, how can you best describe its behavior?
You can assume number
is a positive integer and isPrime(x)
returns true if x
is prime and false otherwise.
list ← []
i ← 1
REPEAT number TIMES
{
IF (isPrime(i))
{
APPEND(list, i)
}
i ← i + 1
}
DISPLAY LENGTH(list)
A. This code displays the total number of prime numbers between 1 and number
✅
B. This code displays a list of all of the prime numbers between 1 and number
C. This code displays the sum of the prime numbers between 1 and number
D. This code results in an error
Question: 4
The AP Exam uses the following format to remove an item from a list and to access the element at a specific index in a list.
REMOVE(aList, i)
aList[i]
Given the following Mystery
procedure, how can you best describe the result it returns?
PROCEDURE Mystery (list, target)
{
length ← LENGTH(list)
i ← 1
REPEAT length TIMES
{
IF (list[i] = target)
{
REMOVE(list, i)
}
ELSE
{
i ← i + 1
}
}
RETURN(list)
}
A. This procedure returns the list without the first instance of the given target
B. This procedure returns the list without any instance of the given target
✅
C. This procedure returns the list where each instance of the given target
is replaced by the index.
D. This procedure returns the list where each instance of the given target
is replaced by the index.
E. This procedure returns the number of times the target
appears in the list
公众号:AI悦创【二维码】
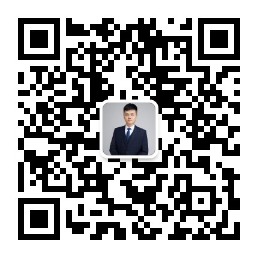
AI悦创·编程一对一
AI悦创·推出辅导班啦,包括「Python 语言辅导班、C++ 辅导班、java 辅导班、算法/数据结构辅导班、少儿编程、pygame 游戏开发、Web、Linux」,全部都是一对一教学:一对一辅导 + 一对一答疑 + 布置作业 + 项目实践等。当然,还有线下线上摄影课程、Photoshop、Premiere 一对一教学、QQ、微信在线,随时响应!微信:Jiabcdefh
C++ 信息奥赛题解,长期更新!长期招收一对一中小学信息奥赛集训,莆田、厦门地区有机会线下上门,其他地区线上。微信:Jiabcdefh
方法一:QQ
方法二:微信:Jiabcdefh
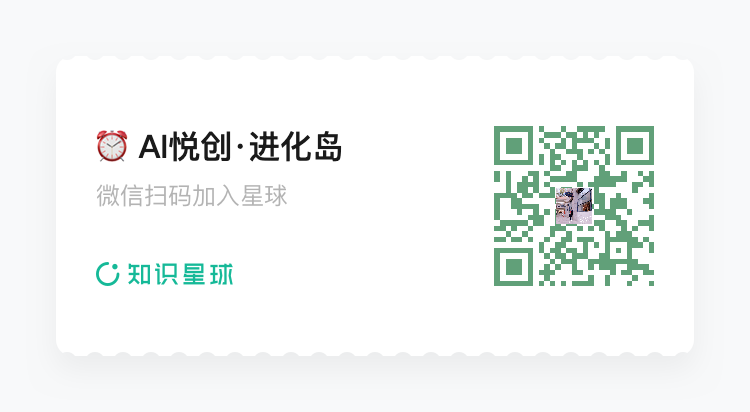
- 0
- 0
- 0
- 0
- 0
- 0