06-NYU Tandon School of Engineering「Homework 04」
NYU Tandon School of Engineering
纽约大学坦顿工程学院
Due: 1159pm, Thursday, March 3rd, 2023
截止时间:2023年3月3日星期四晚上11 ~ 59分
Submission instructions
提交说明
- You should submit your homework on Gradescope.
你应该在Gradescope上提交作业。
For this assignment you should turn in 4 separate .py files named according to the following pattern:
hw4_q1.py, hw4_q2.py, etc.
对于这个任务,你应该提交4个独立的.py文件,按照以下模式命名:
Hw4_q1.py, hw4_q2.py等。
- Each Python file you submit should contain a header comment block as follows:
你提交的每个Python文件都应该包含一个头注释块,如下所示:
"""
Author: [Your name here]
Assignment / Part: HW4 - Q1 (etc.)
Date due: 2023-03-02, 11:59pm
I pledge that I have completed this assignment without
collaborating with anyone else, in conformance with the
NYU School of Engineering Policies and Procedures on
Academic Misconduct.
"""
No late submissions will be accepted.
逾期提交的资料恕不受理。
REMINDER: Do not use any Python structures that we have not learned in class.
提醒:不要使用任何我们在课堂上没有学过的Python结构。
For this specific assignment, you may use everything we have learned up to, and including, variables, types, mathematical and boolean expressions, user IO (i.e. print() and input()), number systems, and the math /random modules, selection statements (i.e. if, elif, else), and for- and while-loops. Please reach out to us if you're at all unsure about any instruction or whether a Python structure is or is not allowed.
对于这个特定的赋值,你可以使用我们学到的所有东西,包括变量、类型、数学和布尔表达式、用户IO(即print()和input())、数字系统和数学/随机模块、选择语句(即if、elif、else)以及For -和while-循环。如果您不确定任何指令或Python结构是否被允许,请与我们联系。
Do not use, for example, user-defined functions (except for main() if your instructor has covered it during lecture), string methods, file i/o, exception handling, dictionaries, lists, tuples, and/or object-oriented programming.
例如,不要使用用户定义的函数(main()除外,如果你的导师在课堂上讲过)、字符串方法、文件i/o、异常处理、字典、列表、元组和/或面向对象编程。
Failure to abide by any of these instructions will make your submission subject to point deductions.
如不遵守上述任何一项规定,您的投稿将被扣分。
Problems
- (s)rewoP ehT toG ev'I (hw4_q1.py)
- Tamako Market (hw4_q2.py)
- Must be funny in the rich man's world (hw4_q3.py)
- A Little Imagination Goes A Long Way In Fez (hw4_q4.py)
Question 1: (s)rewoP ehT toG ev'I
# -*- coding: utf-8 -*-
# @Time : 2023/3/3 00:31
# @Author : AI悦创
# @FileName: q1.py
# @Software: PyCharm
# @Blog :https://bornforthis.cn/
condition = False
base = ""
while not condition:
base = input("Please enter a positive integer to serve as the base:")
if not base.isdigit():
if base.count(".") == 1:
print("Invalid value for the base (" + str(base) + ").", end="")
elif not int(base) > 0:
print("Invalid value for the base (" + str(base) + ").", end="")
elif not int(base) > 0: # 要不要 >= ?
print("Invalid value for the base (" + str(base) + ").", end="")
else:
base = int(base)
condition = True
condition_power = False
power = ""
while not condition_power:
power = input("Please enter a positive integer to serve as the highest power:")
if not power.isdigit():
if power.count(".") == 1:
print("Invalid value for the power (" + str(power) + ").", end="")
elif not int(power) > 0:
print("Invalid value for the power (" + str(power) + ").", end="")
elif not int(power) > 0: # 要不要 >= ?
print("Invalid value for the power (" + str(power) + ").", end="")
else:
power = int(power)
condition_power = True
if power % 2 == 1:
power -= 1
for p in range(power, -1, -2):
print(str(base) + " ^ " + str(p) + " = " + str(base ** p))
# -*- coding: utf-8 -*-
# @Time : 2023/3/3 00:31
# @Author : AI悦创
# @FileName: q1.py
# @Software: PyCharm
# @Blog :https://bornforthis.cn/
condition = False
base = ""
while not condition:
base = input("Please enter a positive integer to serve as the base:")
if "." in base:
print("Invalid value for the base (" + str(base) + ").", end="")
elif not int(base) > 0:
print("Invalid value for the base (" + str(base) + ").", end="")
else:
base = int(base)
condition = True
condition_power = False
power = ""
while not condition_power:
power = input("Please enter a positive integer to serve as the highest power:")
if "." in power:
print("Invalid value for the power (" + str(power) + ").", end="")
elif not int(power) > 0:
print("Invalid value for the power (" + str(power) + ").", end="")
else:
power = int(power)
condition_power = True
if power % 2 == 1:
power -= 1
for p in range(power, -1, -2):
print(str(base) + " ^ " + str(p) + " = " + str(base ** p))
Question 2: Tamako Market
# Define constants for the conversion factor of grams to cups
GRAMS_PER_CUP = 220.0
# Get user input for the amount of each ingredient in grams
mochiko_grams = float(input("Enter an amount (g) of mochiko: "))
sugar_grams = float(input("Enter an amount (g) of sugar: "))
cornstarch_grams = float(input("Enter an amount (g) of cornstarch: "))
anko_grams = float(input("Enter an amount (g) of anko: "))
# Convert the amount of each ingredient from grams to cups
mochiko_cups = mochiko_grams / GRAMS_PER_CUP
sugar_cups = sugar_grams / GRAMS_PER_CUP
cornstarch_cups = cornstarch_grams / GRAMS_PER_CUP
anko_cups = anko_grams / GRAMS_PER_CUP
# Calculate the number of batches of daifuku mochi that can be made
batches = min(mochiko_cups/3, sugar_cups/1.5, cornstarch_cups/2, anko_cups)
# Print the result
print("With this amount of ingredients, you can make {} batch(es) of 24 mochi.".format(int(batches)))
当用户输入了一定量的麻糬粉、糖、玉米淀粉和红豆馅的克数之后,此 Python 程序会帮助计算可以用这些配料制作出多少批(每批 24 个)大福麻糬。具体操作步骤如下:
- 用户将输入一定量的麻糬粉、糖、玉米淀粉和红豆馅,单位为克。
- 程序将把克数转换为杯数(1 杯 = 220 克)。
- 程序将计算可以用这些配料制作出多少批大福麻糬。
该程序使用 input()
函数获取用户输入。然后,它将使用定义的常量 GRAMS_PER_CUP
(每杯克数)将每个成分的克数转换为杯数,并使用最小值函数计算出可以制作的麻糬批次数。最后,程序使用字符串格式化将结果打印输出。
Question 3: Must be funny in the rich man's world
# -*- coding: utf-8 -*-
# @Time : 2023/3/3 00:56
# @Author : AI悦创
# @FileName: q3.py
# @Software: PyCharm
# @Blog :https://bornforthis.cn/
condition = False
players_num = 0
while not condition:
players_num = input("How many players played this round? ")
if not players_num.isdigit():
print("Invalid input.", end="")
else:
players_num = int(players_num)
condition = True
people = 1 # 表示第几个人
# condition = False
max_value = 0
index = 0
while players_num >= people: # 也可以使用 range 实现
property_total = 0
details_condition = False
while not details_condition:
property = input("Enter the value of a property/asset, or DONE to finish:") # 但是可以假设用户永远不会输入浮点值
if property == "DONE":
details_condition = True
elif float(property) > 0:
property_total += float(property)
else:
print("Invalid input. ") # else 或许不需要写
print("Player {} has {:.2f} dollars.".format(people, property_total))
if property_total > max_value:
max_value = property_total
index = people
people += 1
print("Congratulations, player {}! You won with ${}!".format(index, max_value))
# -*- coding: utf-8 -*-
# @Time : 2023/3/3 00:56
# @Author : AI悦创
# @FileName: q3.py
# @Software: PyCharm
# @Blog :https://bornforthis.cn/
condition = False
players_num = 0
while not condition:
players_num = input("How many players played this round? ")
if "." in players_num:
print("Invalid input.", end="")
else:
players_num = int(players_num)
condition = True
people = 1 # 表示第几个人
# condition = False
max_value = 0
index = 0
while players_num >= people: # 也可以使用 range 实现
property_total = 0
details_condition = False
while not details_condition:
property = input("Enter the value of a property/asset, or DONE to finish:") # 但是可以假设用户永远不会输入浮点值
if property == "DONE":
details_condition = True
elif float(property) > 0:
property_total += float(property)
else:
print("Invalid input. ") # else 或许不需要写
print("Player {} has {:.2f} dollars.".format(people, property_total))
if property_total > max_value:
max_value = property_total
index = people
people += 1
print("Congratulations, player {}! You won with ${}!".format(index, max_value))
Problem 4: A Little Imagination Goes A Long Way In Fez
# -*- coding: utf-8 -*-
# @Time : 2023/3/3 01:34
# @Author : AI悦创
# @FileName: q4-1.py
# @Software: PyCharm
# @Blog :https://bornforthis.cn/
from turtle import *
def createSquare():
forward(150)
for i in range(3):
right(90)
forward(150)
right(15)
for i in range(36):
createSquare()
exitonclick()
# -*- coding: utf-8 -*-
# @Time : 2023/3/3 07:41
# @Author : AI悦创
# @FileName: q4.py
# @Software: PyCharm
# @Blog :https://bornforthis.cn/
# Moroccan Mosaic using Python Turtle - www.101computing.net/morroccan-mosaic/
import turtle
myPen = turtle.Turtle()
numberOfIterations = 16 # 循环次数
size = 100 # 线条长度
myPen.speed(1000) # 设定乌龟的速度
# 通过重复和旋转多边形形状绘制马赛克的程序。
def main(numberOfSides):
for i in range(0, numberOfIterations):
for j in range(0, numberOfSides):
myPen.forward(size)
myPen.left(360 / numberOfSides)
myPen.left(360 / numberOfIterations)
# 主程序从这里开始
# 马赛克 #1
user_input = int(input("请输入你要实现的多边形:"))
main(user_input)
turtle.done()
# -*- coding: utf-8 -*-
# @Time : 2023/3/3 07:41
# @Author : AI悦创
# @FileName: q4.py
# @Software: PyCharm
# @Blog :https://bornforthis.cn/
# Moroccan Mosaic using Python Turtle - www.101computing.net/morroccan-mosaic/
import turtle
myPen = turtle.Turtle()
numberOfIterations = 16 # 循环次数
size = 100 # 线条长度
myPen.speed(1000) # 设定乌龟的速度
numberOfSides = int(input("请输入你要实现的多边形:"))
# 通过重复和旋转多边形形状绘制马赛克的程序。
def main():
for i in range(0, numberOfIterations):
for j in range(0, numberOfSides):
myPen.forward(size)
myPen.left(360 / numberOfSides)
myPen.left(360 / numberOfIterations)
# 主程序从这里开始
# 马赛克 #1
main()
turtle.done()
公众号:AI悦创【二维码】
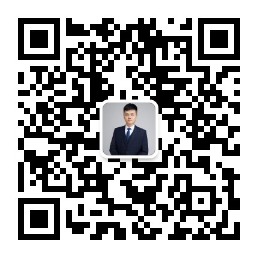
AI悦创·编程一对一
AI悦创·推出辅导班啦,包括「Python 语言辅导班、C++ 辅导班、java 辅导班、算法/数据结构辅导班、少儿编程、pygame 游戏开发、Web、Linux」,全部都是一对一教学:一对一辅导 + 一对一答疑 + 布置作业 + 项目实践等。当然,还有线下线上摄影课程、Photoshop、Premiere 一对一教学、QQ、微信在线,随时响应!微信:Jiabcdefh
C++ 信息奥赛题解,长期更新!长期招收一对一中小学信息奥赛集训,莆田、厦门地区有机会线下上门,其他地区线上。微信:Jiabcdefh
方法一:QQ
方法二:微信:Jiabcdefh
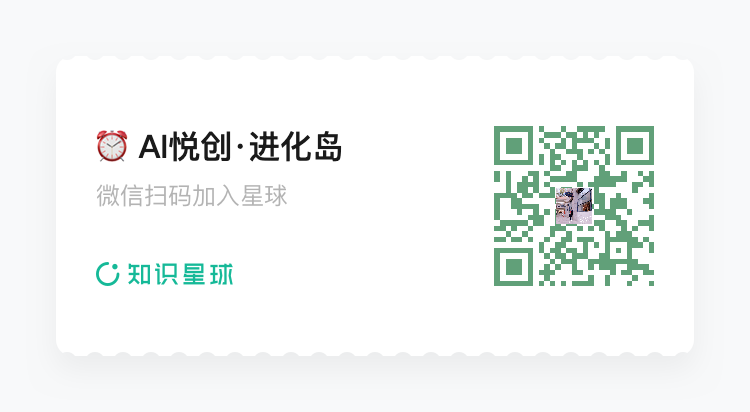
- 0
- 0
- 0
- 0
- 0
- 0