「1月」unique Python辅导
Question 4
问题描述
我按照他的书做了三个小的测试程序,最后这个主程序报错,他说我没有定义 formatted
长图
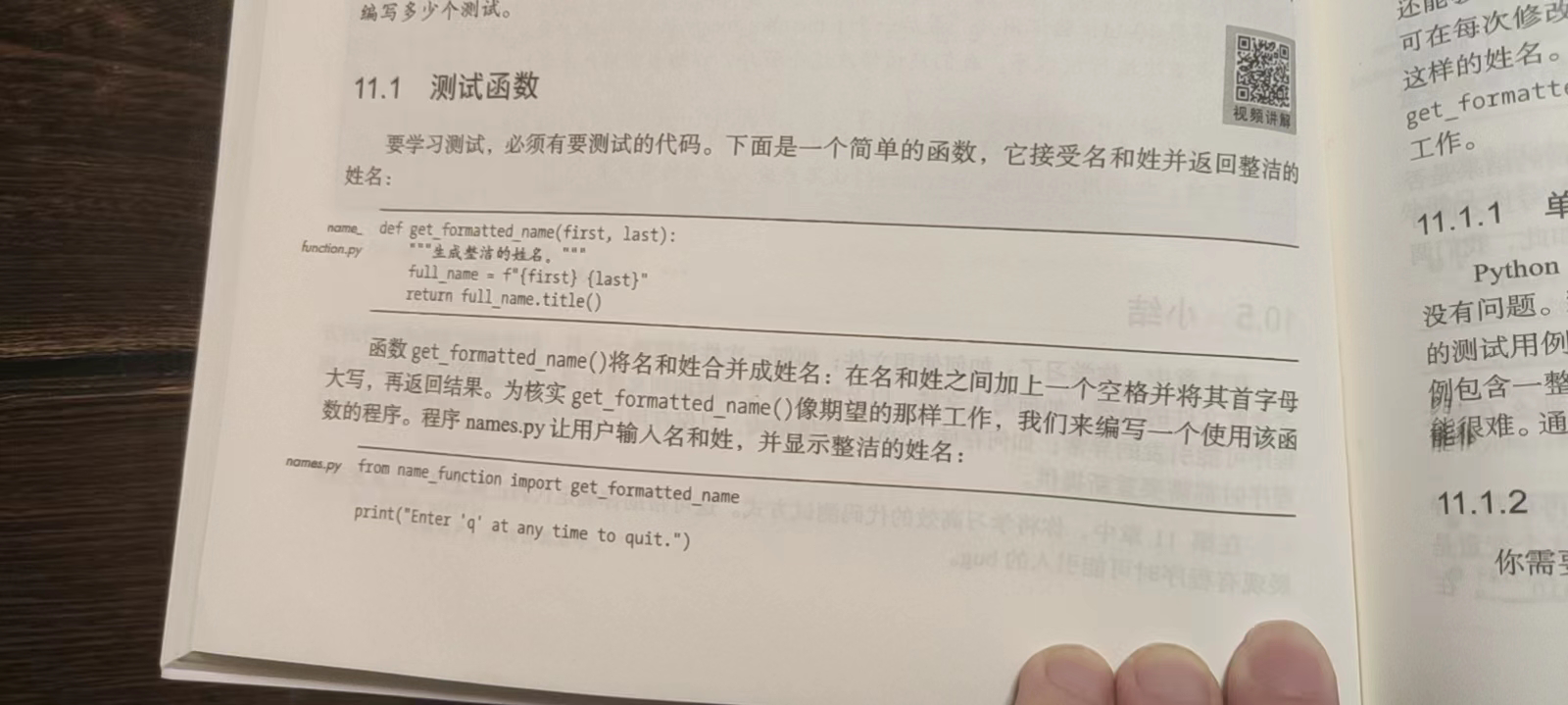
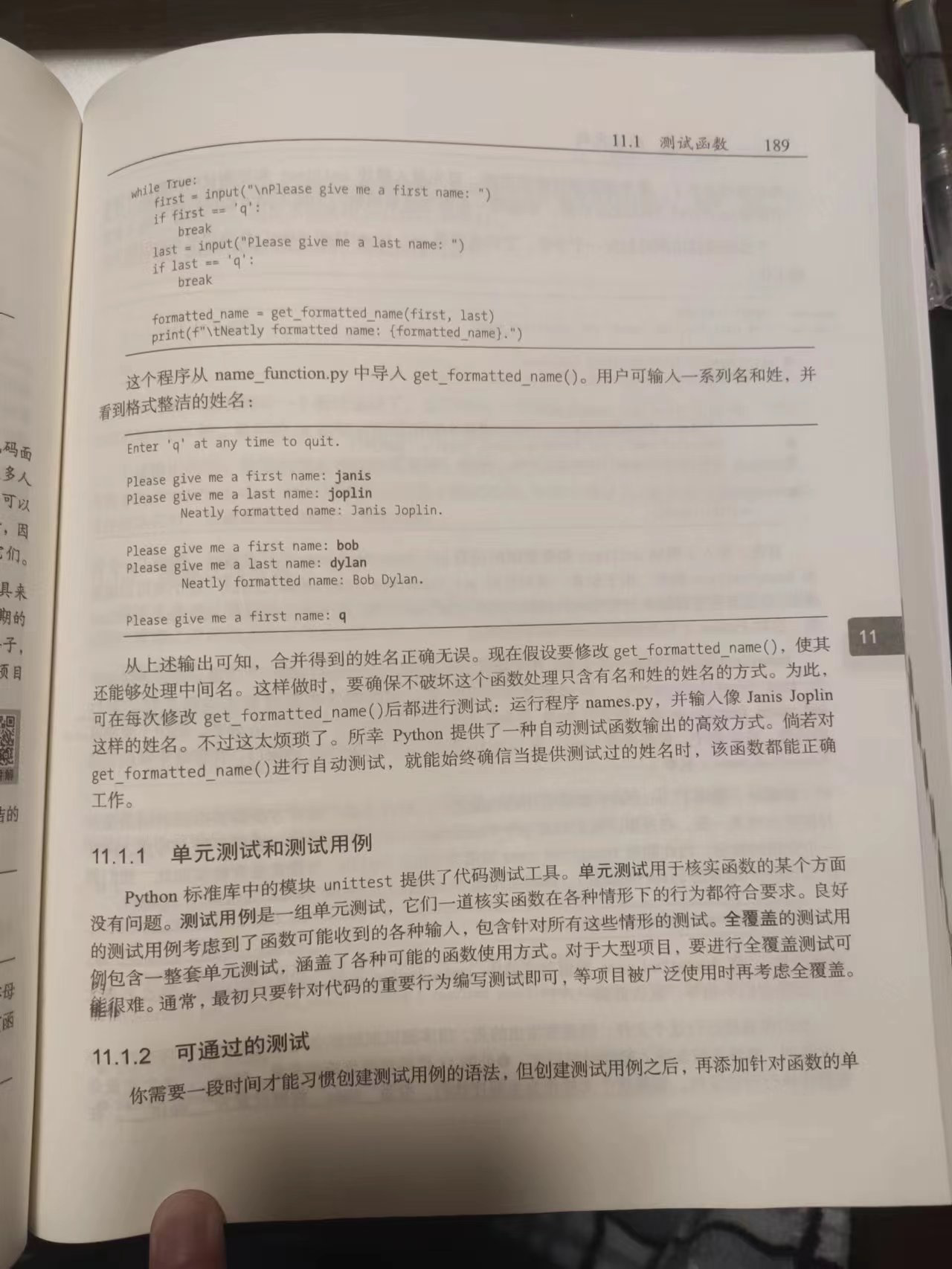

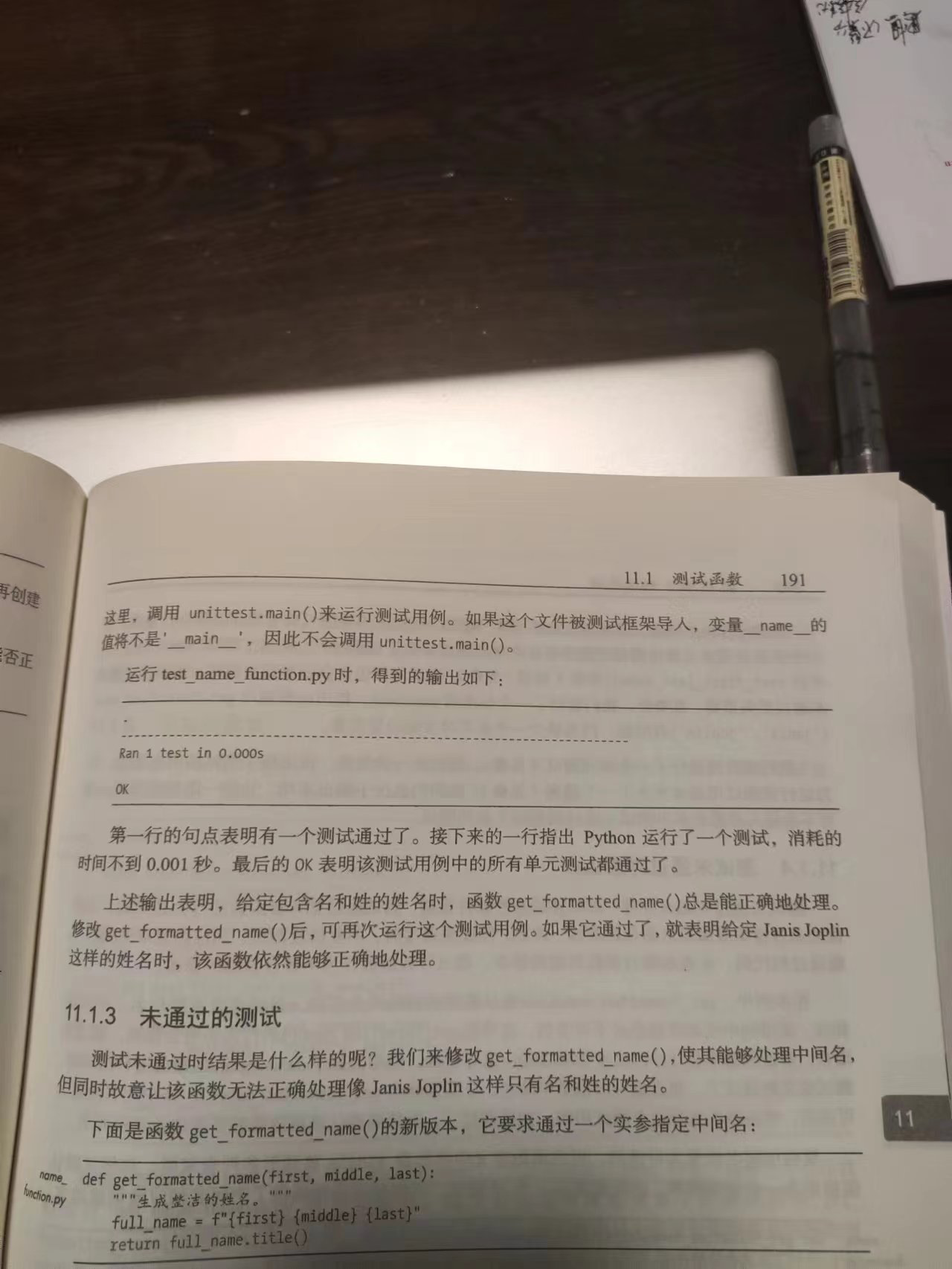
长图
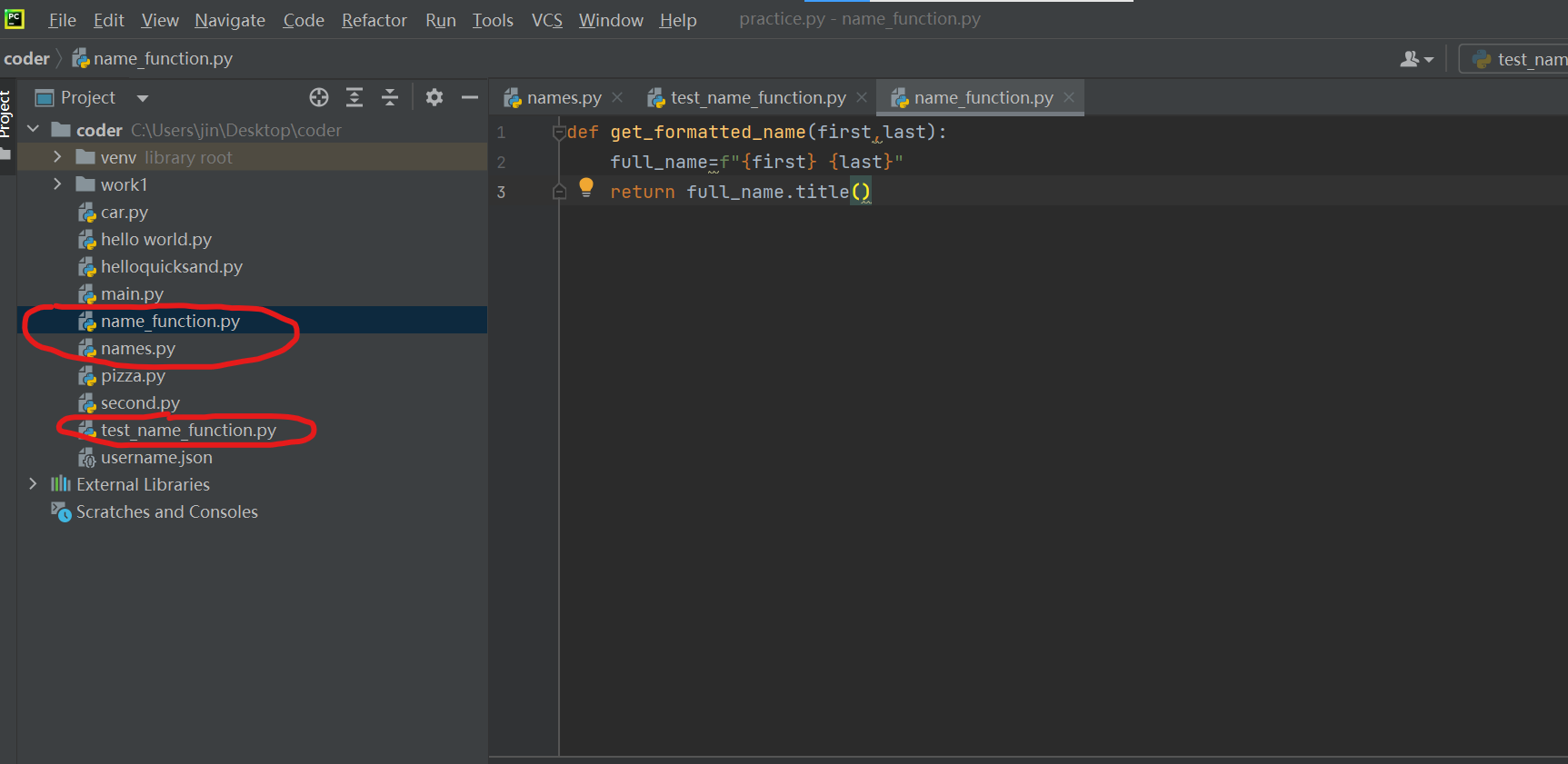
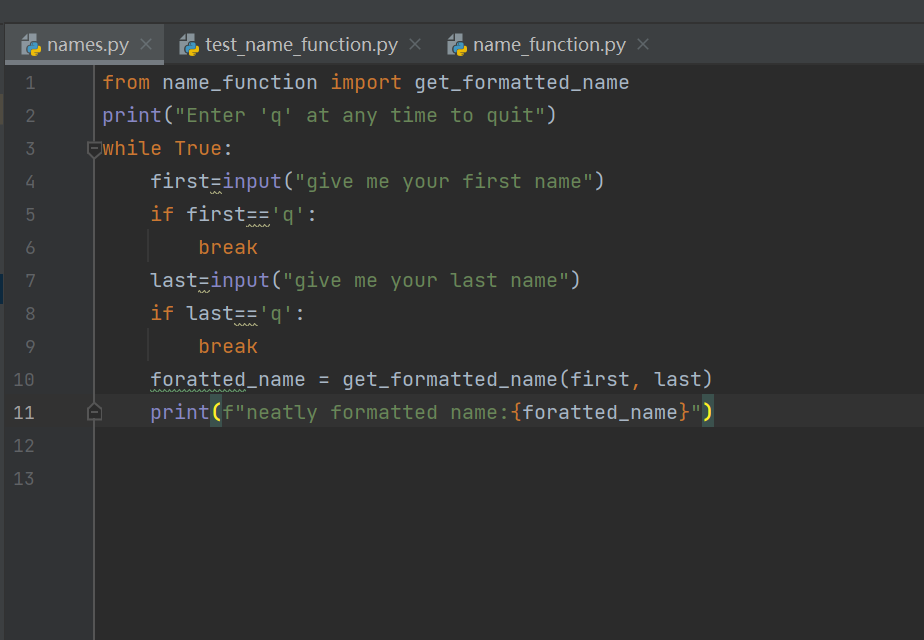

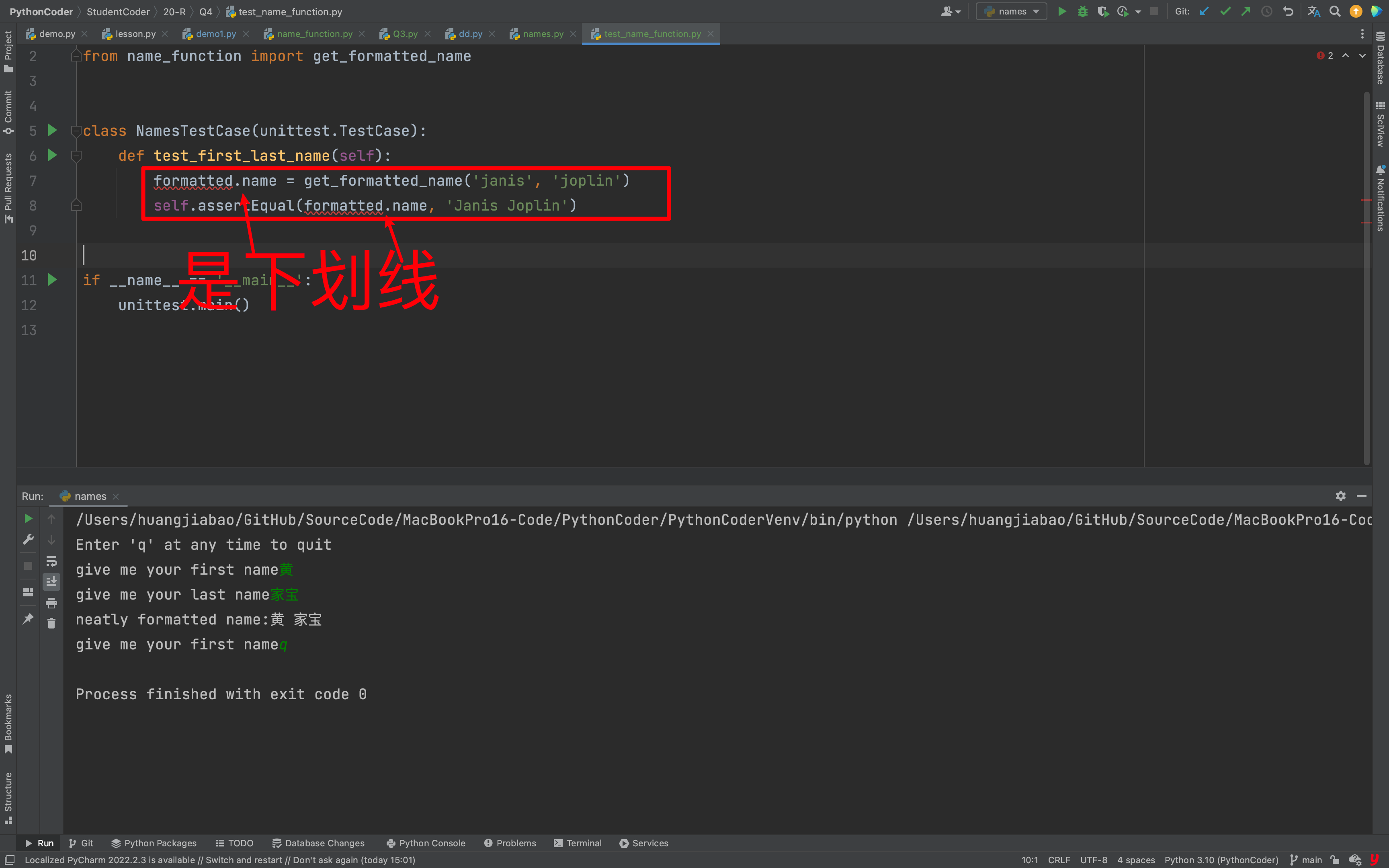
# -*- coding: utf-8 -*-
# @Time : 2023/1/4 20:06
# @Author : AI悦创
# @FileName: name_function.py
# @Software: PyCharm
# @Blog :https://bornforthis.cn/
def get_formatted_name(first, last):
"""生成整洁的姓名"""
full_name = f"{first} {last}"
return full_name.title()
from name_function import get_formatted_name
print("Enter 'q' at any time to quit")
while True:
first = input("give me your first name")
if first == 'q':
break
last = input("give me your last name")
if last == 'q':
break
formatted_name = get_formatted_name(first, last)
print(f"neatly formatted name:{formatted_name}")
import unittest
from name_function import get_formatted_name
class NamesTestCase(unittest.TestCase):
def test_first_last_name(self):
formatted_name = get_formatted_name('janis', 'joplin')
self.assertEqual(formatted_name, 'Janis Joplin')
if __name__ == '__main__':
unittest.main()
Question 5
问题描述
本来测试不需要输入,他直接会告诉你行不行,因为类里面已经输入了english。但是我的代码要求输入,而且输啥都行,然后测试结果永远是 ok
长图



之前都没毛病,怎么学到这里一个个报错😂

practice 储存的函数,pratice2 是测试
问题在于 if __name__ == '__main__':
:
class AnonymousSurvey:
def __init__(self, question):
self.question = question
self.answer = []
def show_question(self):
print(self.question)
def store_respond(self, new_answer):
self.answer.append(new_answer) # 列表添加数据
def show_result(self):
print("Survey answer")
for respond in self.answer:
print(f"\n-{respond}")
if __name__ == '__main__':
question = "What language did you learn"
my_survey = AnonymousSurvey(question) # 类的实例化
my_survey.show_question() # 显示问题
while True:
answer = input("language")
if answer == 'q':
break
my_survey.store_respond(answer) # 把用户回答添加到列表
my_survey.show_result() # 输出用户的回答
if __name__ == '__main__'
我们再来讲讲 if __name__ == '__main__'
,这个我们经常看到的写法。
Python 是脚本语言,和 C++、Java 最大的不同在于,不需要显式提供 main()
函数入口。如果你有 C++、Java 等语言经验,应该对 main() {}
这样的结构很熟悉吧?
不过,既然 Python 可以直接写代码,if __name__ == '__main__'
这样的写法,除了能让 Python 代码更好看(更像 C++ )外,还有什么好处吗?
项目结构如下:
.
├── utils.py
├── utils_with_main.py
├── main.py
└── main_2.py
# utils.py
def get_sum(a, b):
return a + b
print('testing')
print('{} + {} = {}'.format(1, 2, get_sum(1, 2)))
# utils_with_main.py
def get_sum(a, b):
return a + b
if __name__ == '__main__':
print('testing')
print('{} + {} = {}'.format(1, 2, get_sum(1, 2)))
# main.py
from utils import get_sum
print('get_sum: ', get_sum(1, 2))
########## 输出 ##########
testing
1 + 2 = 3
get_sum: 3
# main_2.py
from utils_with_main import get_sum
print('get_sum: ', get_sum(1, 2))
########## 输出 ##########
get_sum_2: 3
看到这个项目结构,你就很清晰了吧。
import 在导入文件的时候,会自动把所有暴露在外面的代码全都执行一遍。因此,如果你要把一个东西封装成模块,又想让它可以执行的话,你必须将要执行的代码放在 if __name__ == '__main__'
下面。
为什么呢?其实,__name__
作为 Python 的魔术内置参数,本质上是模块对象的一个属性。我们使用 import 语句时,__name__
就会被赋值为该模块的名字,自然就不等于 __main__
了。更深的原理我就不做过多介绍了,你只需要明白这个知识点即可。

还是刚刚那个代码有两个点确认一下
就是这个黄的是 testanonymoussurvey 类继承了 unittest,他是自动运行的是吧
因为之前的类的代码后面都会跟着几句别的代码,我的理解就是别的代码使类运行但类本身不会运行
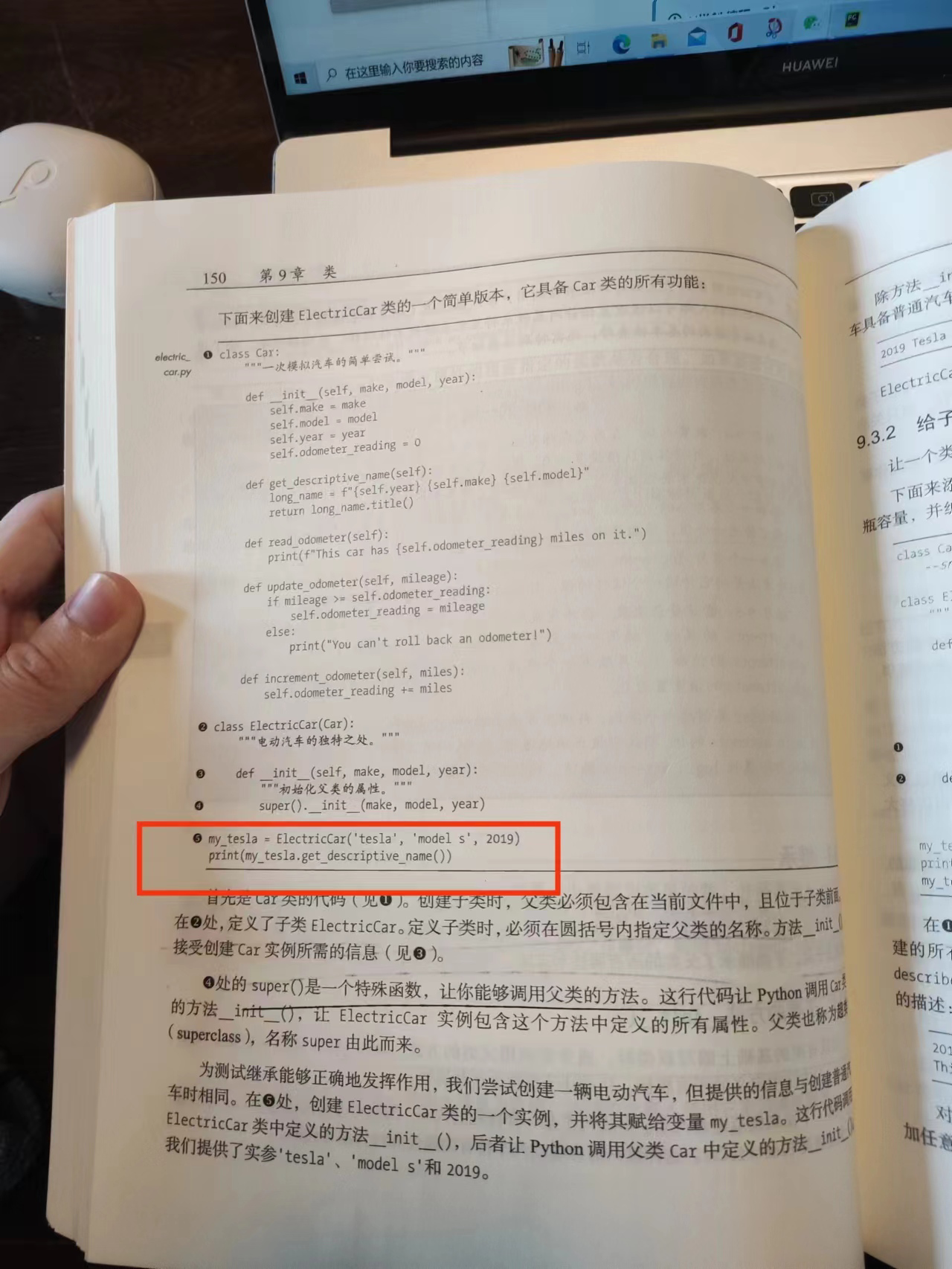
就比如这个创建电动汽车的简单代码,我的理解就是下面红框的让他运行,他本身没有输出
然后这个红框的我其实不太清楚 unittest.main
这个函数会不会运行,又是啥意思
- 就是这个黄的是 testanonymoussurvey 类继承了 unittest,他是自动运行的是吧
Answer
具体来说是 TestAnonymousSurvey 类,继承 unittest.TestCase
。
- 就比如这个创建电动汽车的简单代码,我的理解就是下面红框的让他运行,他本身没有输出
Answer
有没有输出都得看具体的代码,不是只看之前的代码没有输出,一概而论就说后面的代码也应该没有输出。编程是变化的,不是一层不变的,就算找规律,那我们是不是也得多看几组甚至是更多组代码,来运行、找出规律呢?
- 然后这个红框的我其实不太清楚
unittest.main
这个函数会不会运行,又是啥意思
Answer
会运行。
因为 if __name__ == '__main__':
本身就是程序入口。

Answer
- 就是第一步运行1,第二步运行2对不对
- yes
- 假如没有1,2会不会自动运行
- 假如没有 1,2 不会自动运行
class AnonymousSurvey:
def __init__(self, question):
self.question = question
self.answer = []
def show_question(self):
print(self.question)
def store_respond(self, new_answer):
self.answer.append(new_answer) # 列表添加数据
def show_result(self):
print("Survey answer")
for respond in self.answer:
print(f"\n-{respond}")
question = "What language did you learn"
my_survey = AnonymousSurvey(question) # 类的实例化
my_survey.show_question() # 显示问题
while True:
answer = input("language")
if answer == 'q':
break
my_survey.store_respond(answer) # 把用户回答添加到列表
my_survey.show_result() # 输出用户的回答
import unittest
from practice import AnonymousSurvey
class TestAnonymousSurvey(unittest.TestCase):
def test_respond(self):
question = "What language did you learn"
my_survey = AnonymousSurvey(question)
my_survey.store_respond('English')
# print(my_survey.answer)
self.assertIn('English', my_survey.answer)
if __name__ == '__main__':
unittest.main()
class AnonymousSurvey:
def __init__(self, question):
self.question = question
self.answer = []
def show_question(self):
print(self.question)
def store_respond(self, new_answer):
self.answer.append(new_answer) # 列表添加数据
def show_result(self):
print("Survey answer")
for respond in self.answer:
print(f"\n-{respond}")
if __name__ == '__main__':
question = "What language did you learn"
my_survey = AnonymousSurvey(question) # 类的实例化
my_survey.show_question() # 显示问题
while True:
answer = input("language")
if answer == 'q':
break
my_survey.store_respond(answer) # 把用户回答添加到列表
my_survey.show_result() # 输出用户的回答
Question 6

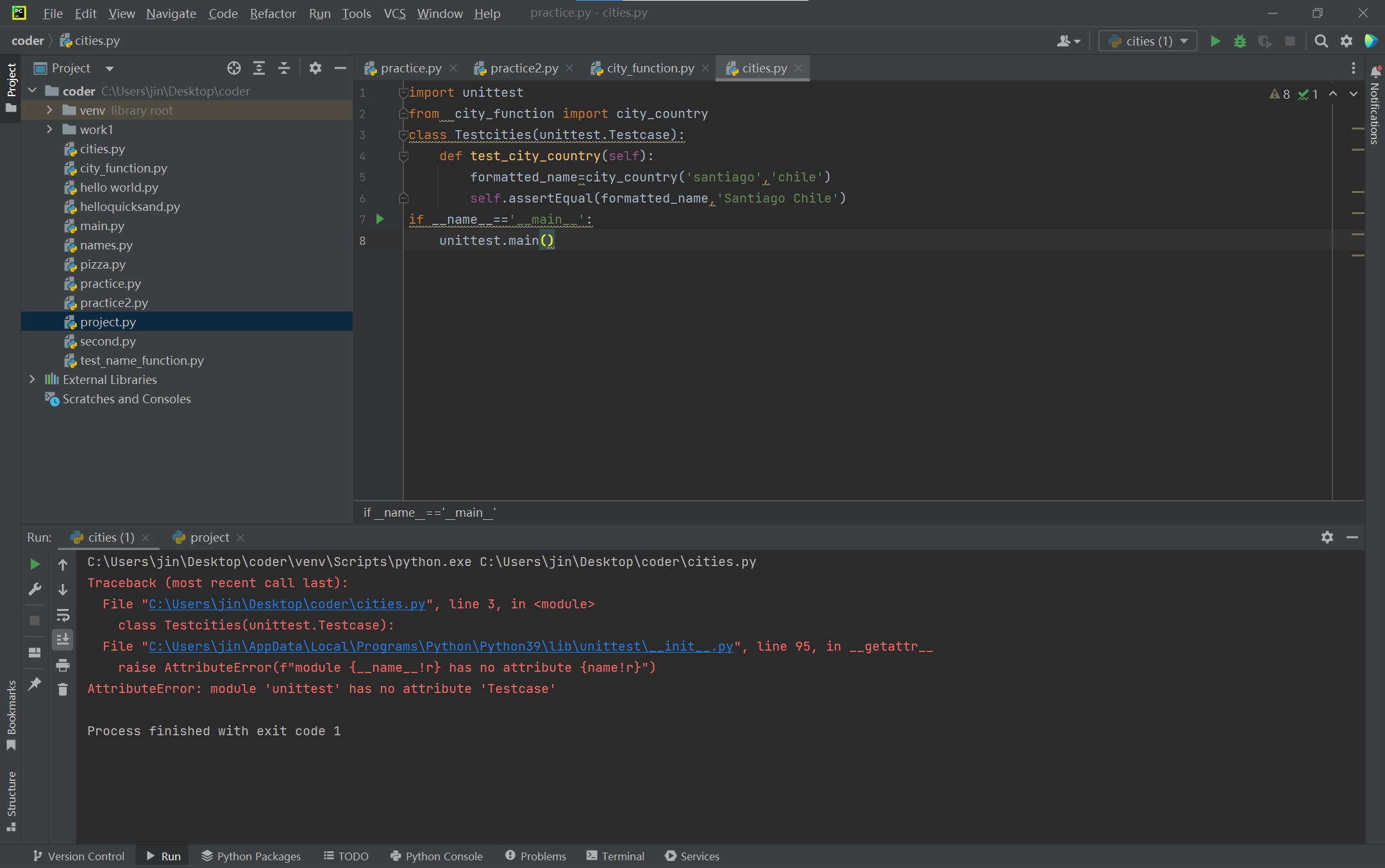
# city_function.py
def city_country(city, country):
hometown = f"{city} {country}"
return hometown.title()
# cities.py
import unittest
from city_function import city_country
class Testcities(unittest.Testcase):
def test_city_country(self):
formatted_name = city_country('santiago', 'chile')
self.assertEqual(formatted_name, 'Santiago Chile')
if __name__ == '__main__':
unittest.main()
这个代码错误:class Testcities(unittest.Testcase):
提示
把 unittest.Testcase
修改成:unittest.TestCase
正确代码:
import unittest
from city_function import city_country
class Testcities(unittest.TestCase):
def test_city_country(self):
formatted_name = city_country('santiago', 'chile')
self.assertEqual(formatted_name, 'Santiago Chile')
if __name__ == '__main__':
unittest.main()
Question 7
好像下载不了。


说明
该问题较为常见,是 pip 在安装时遇到的网络问题。一般来说首先会想到换源,顾名思义:原本默认 Python 请求的都是国外的源文件,但是国内 ban 的原因,需要科学上网或者换源。
- 科学上网也不一定能解决,但是学 IT 不都要翻墙。有需要可以链接:https://bornforthis.cn/vpn.html
- 换源是用如下命令即可「在命令行输入即可」:
pip config set global.index-url https://pypi.douban.com/simple
- 更多换源方法,请参考:https://bornforthis.cn/posts/16.html


我感觉我安装好了,但是他还是说我没安装

说明
你是安装成功了,但是你安装的环境是系统环境,Pycharm 有自己的虚拟环境。所以解决方案如下:
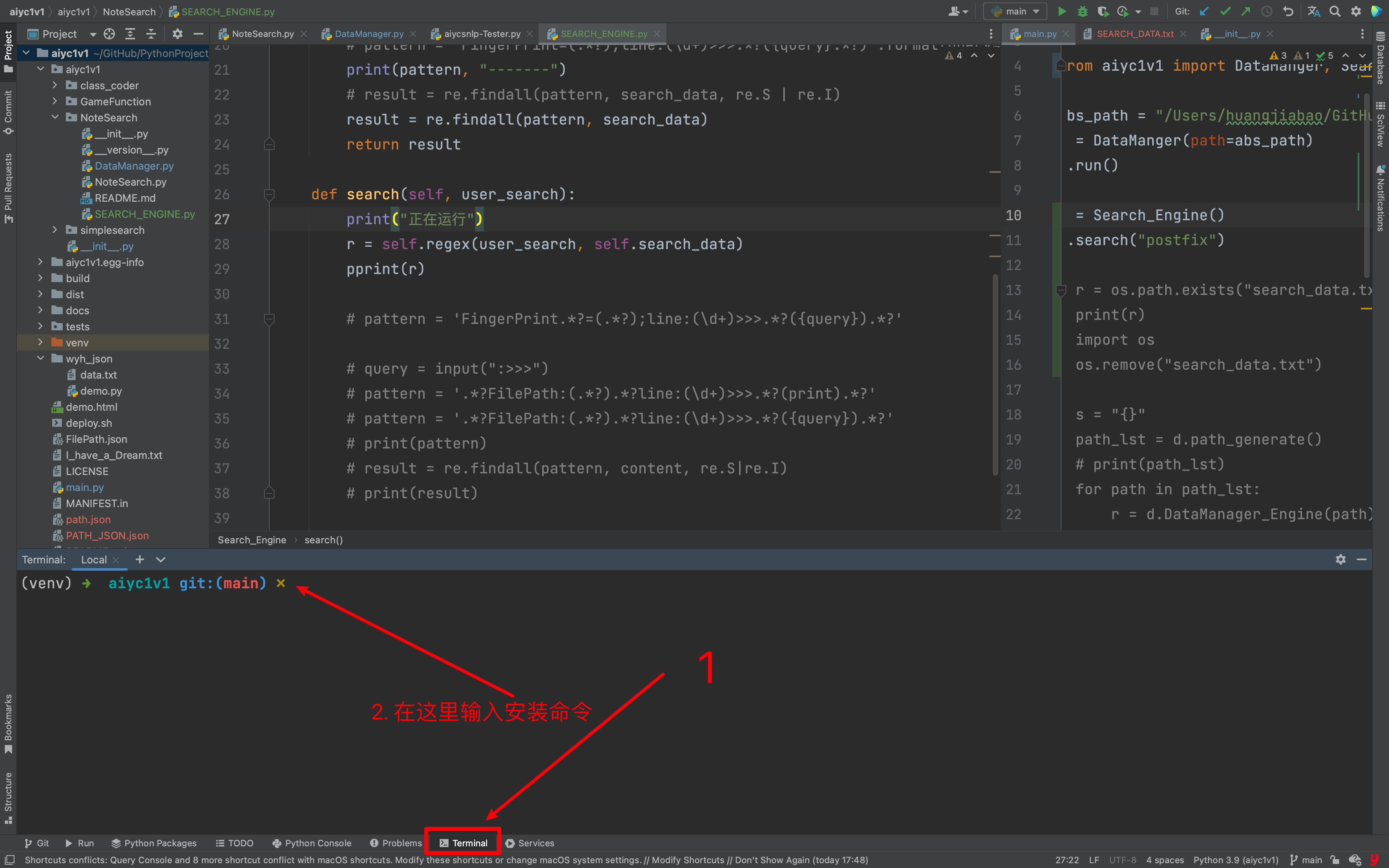
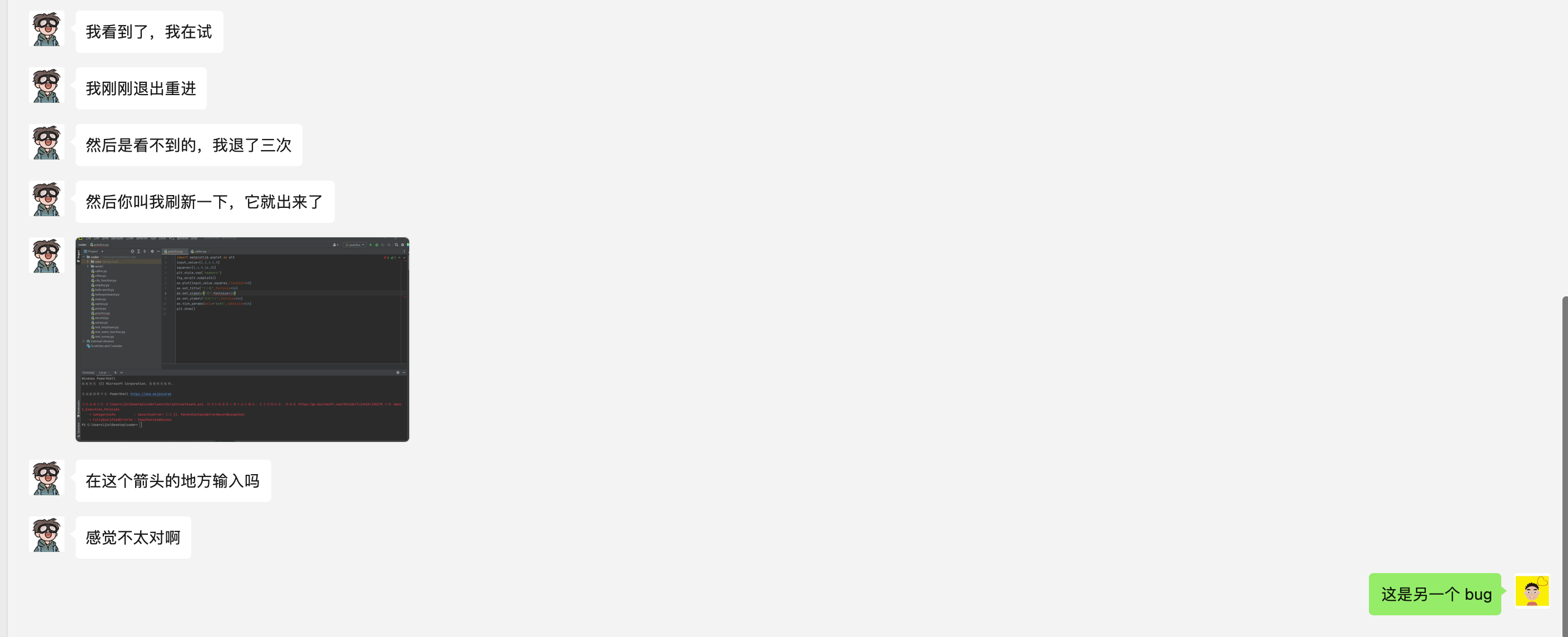
打开 Pycharm 设置界面
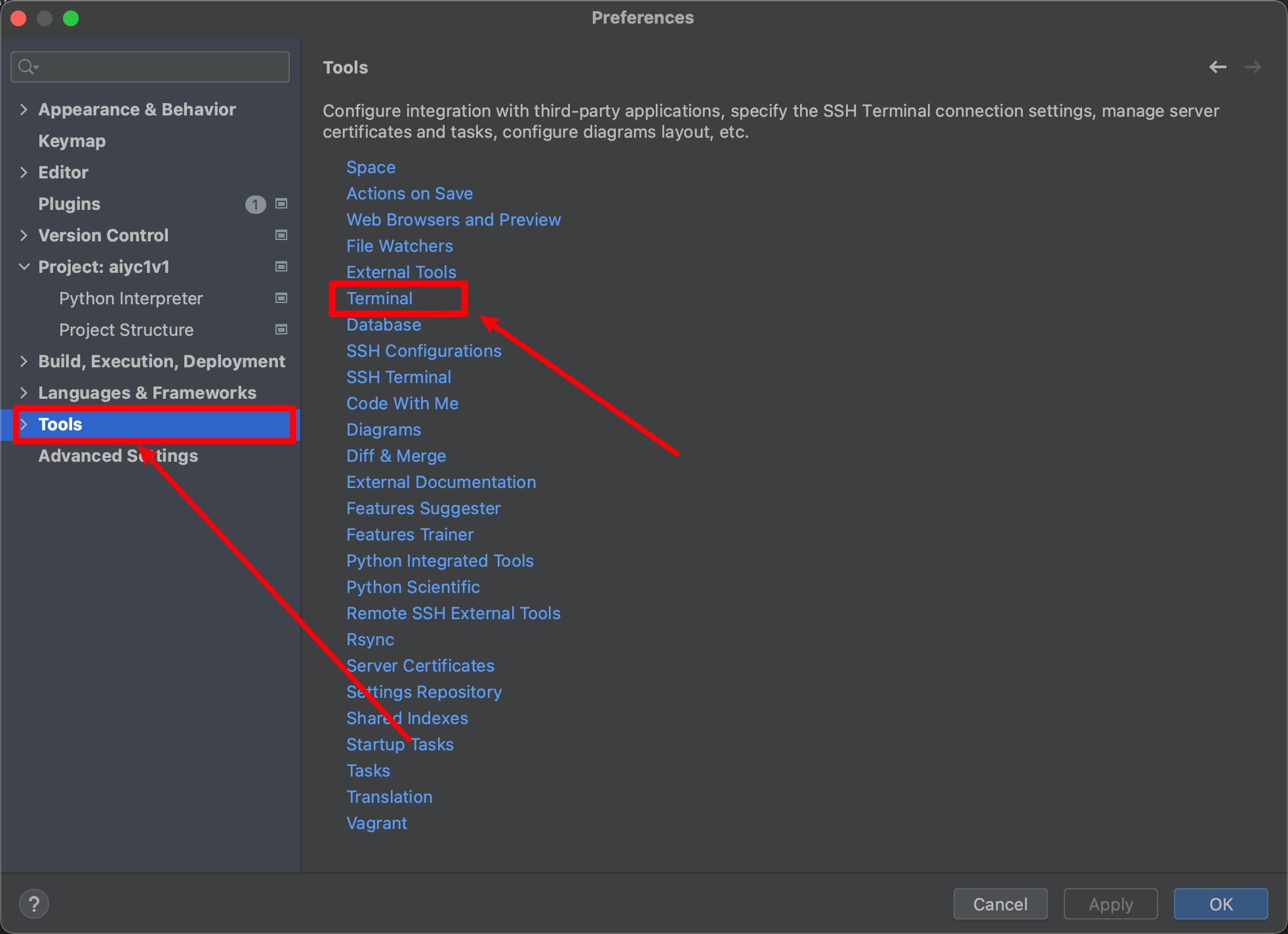
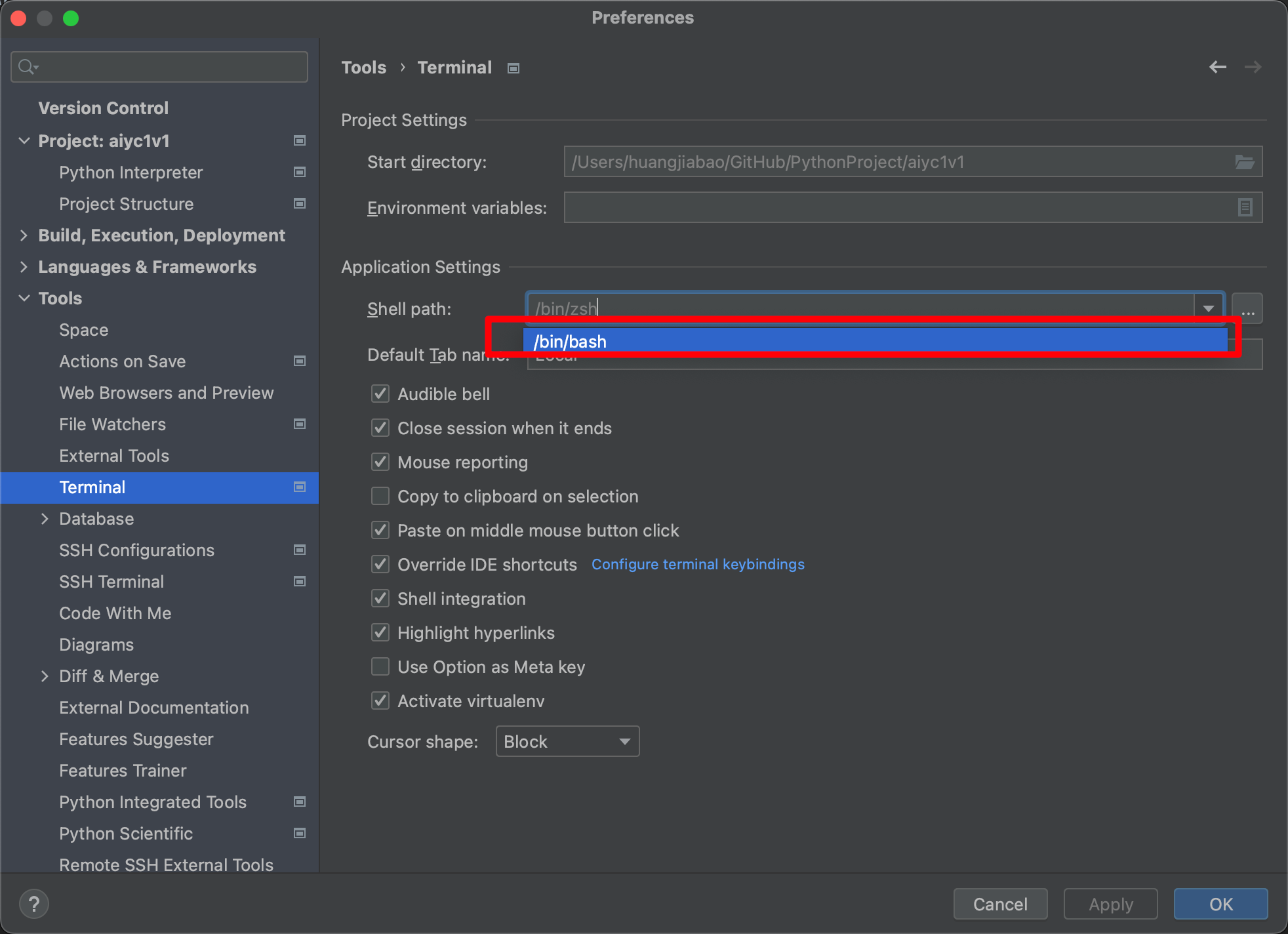
说明
我是 Mac 你选择另外的 cmd 选项即可。
然后记得把 Terminal 关掉「Pycharm 的」,再点开 Pycharm 的 Terminal,再输入安装命令。重复上一个解答方法。
Question 8



描述
这个代码我上次打的时候啥问题也没有,我现在复习了一遍,重新打了一下结果就说这个地方有问题
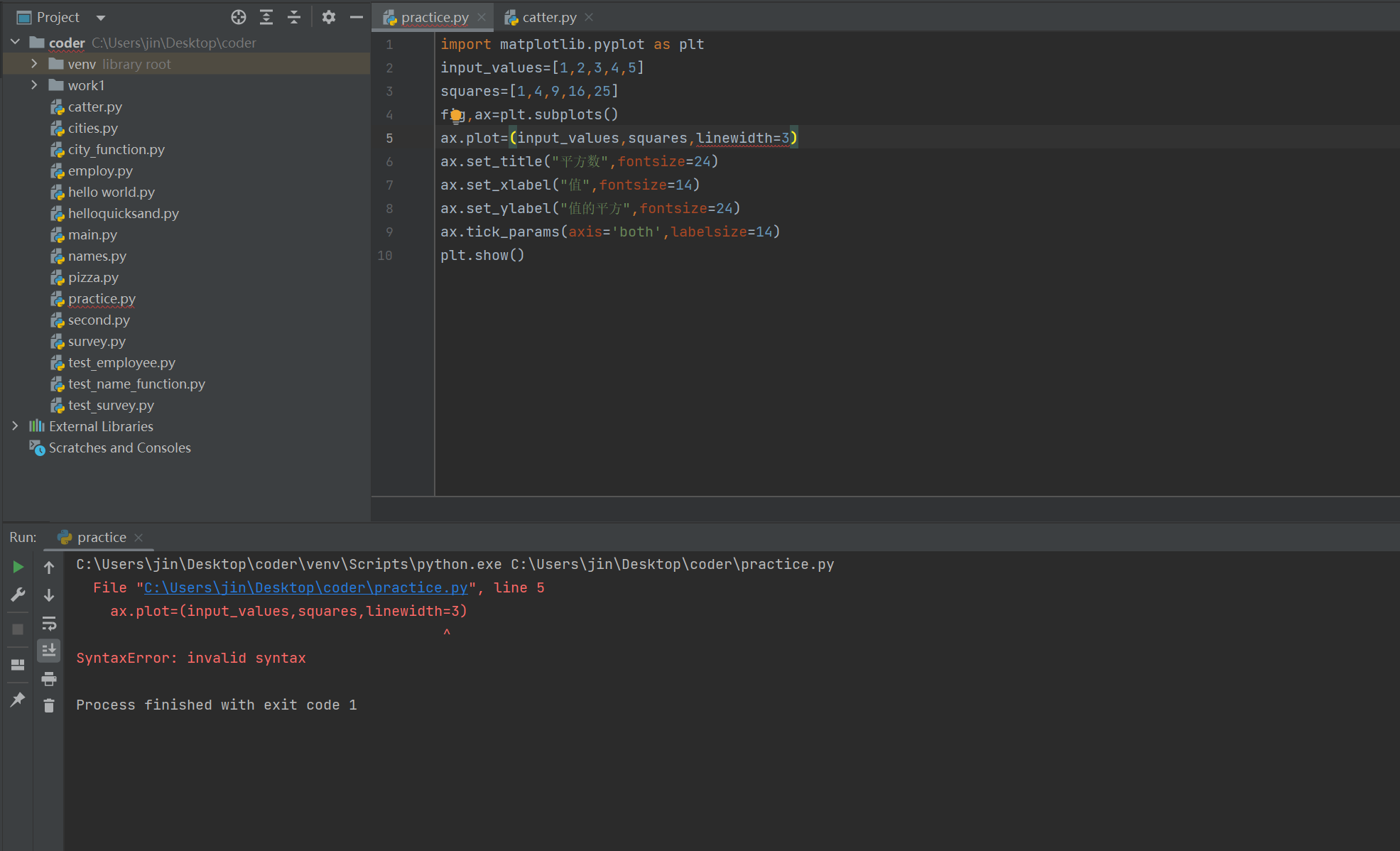
描述
然后我把这个部分给删除了,得到的结果就是这个图但是他旁边的文字怎么都是空格。
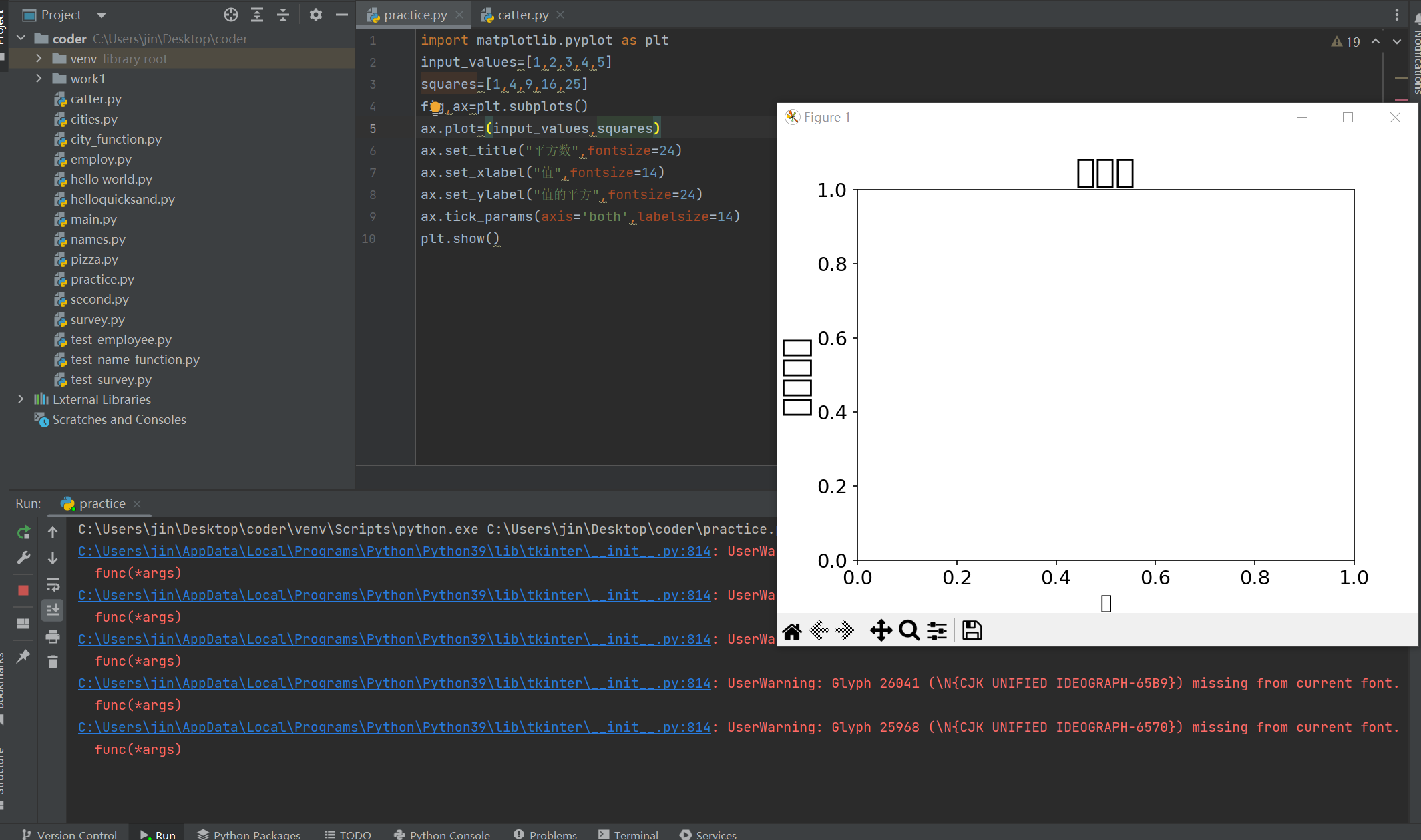
import matplotlib.pyplot as plt
input_values = [1, 2, 3, 4, 5]
squares = [1, 4, 9, 16, 25]
fig, ax = plt.subplots()
ax.plot = (input_values, squares)
ax.set_title("平方数", fontsize=24)
ax.set_xlabel("值", fontsize=14)
ax.set_ylabel("值的平方", fontsize=24)
ax.tick_params(axis='both', labelsize=14)
plt.show()
正确代码:
import matplotlib.pyplot as plt
squares = [1, 4, 9, 16, 25]
fig, ax = plt.subplots()
ax.plot(squares, linewidth=3)
ax.set_title("pf", fontsize=24)
ax.set_xlabel("Value", fontsize=14)
ax.set_ylabel("Value pf", fontsize=14)
ax.tick_params(axis='both', labelsize=14)
plt.show()

说明
你的数据传入错误。
import matplotlib.pyplot as plt
input_values = [1, 2, 3, 4, 5]
squares = [1, 4, 9, 16, 25]
fig, ax = plt.subplots()
# ax.plot = (input_values, squares)
ax.plot(squares, linewidth=3)
ax.set_title("平方数", fontsize=24)
ax.set_xlabel("值", fontsize=14)
ax.set_ylabel("值的平方", fontsize=24)
ax.tick_params(axis='both', labelsize=14)
plt.show()
代码行,高亮部分就是修改部分。
多个数据的话,使用如下:


参考这个方法:https://www.cnblogs.com/hhh5460/p/4323985.html

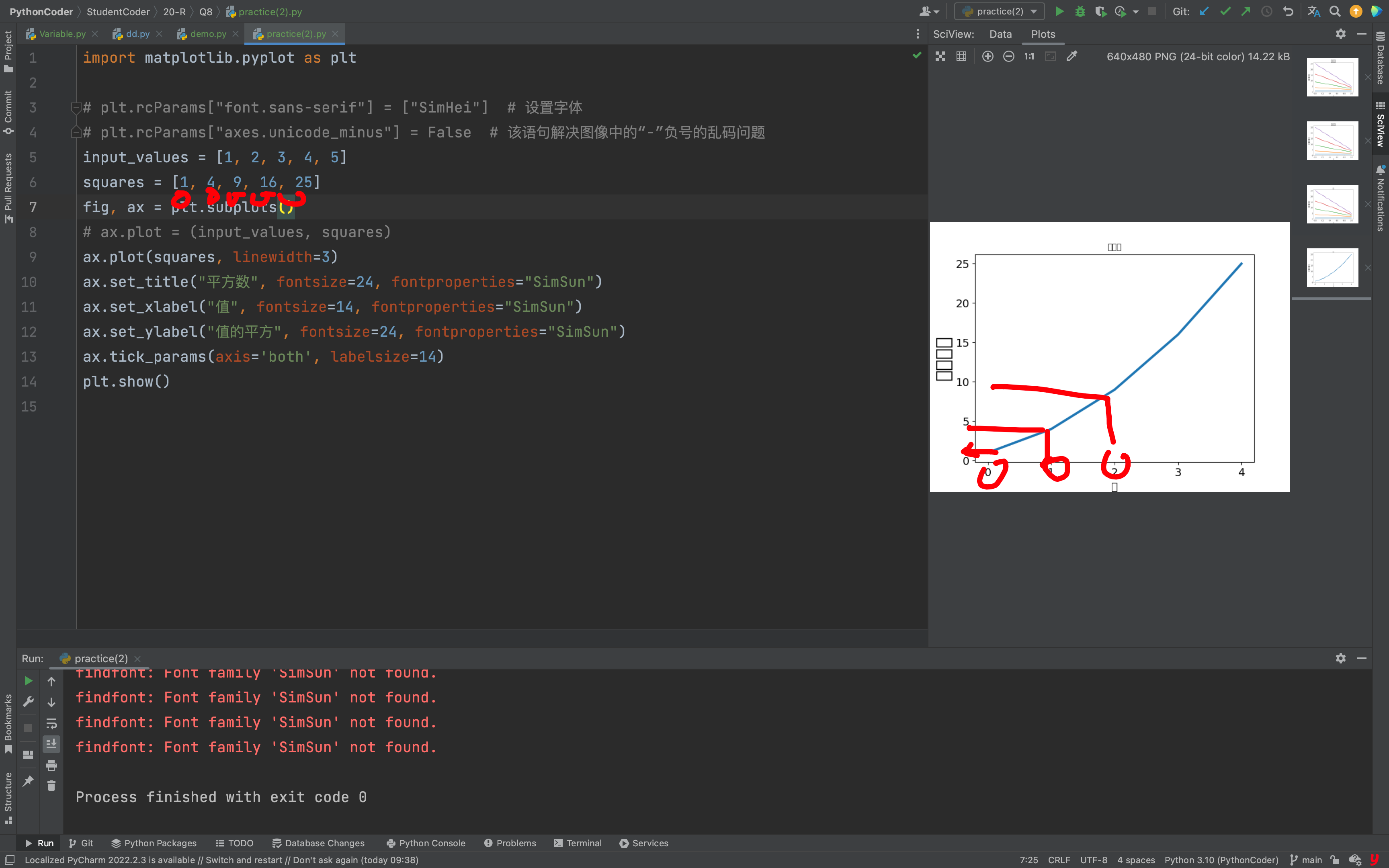

import matplotlib.pyplot as plt
# plt.rcParams["font.sans-serif"] = ["SimHei"] # 设置字体
# plt.rcParams["axes.unicode_minus"] = False # 该语句解决图像中的“-”负号的乱码问题
# input_values = [1, 2, 3, 4, 5]
squares = [1, 4, 9, 16, 25]
fig, ax = plt.subplots()
names = ['GFK', 'SA', 'DA-NBNN', 'DLID', 'DaNN']
x = range(len(names))
# ax.plot = (input_values, squares)
ax.plot(squares, linewidth=3)
ax.set_title("pf", fontsize=24)
ax.set_xlabel("value",fontsize=14)
ax.set_ylabel("value pf", fontsize=24)
plt.xticks(x, names)
ax.tick_params(axis='both', labelsize=14)
plt.show()
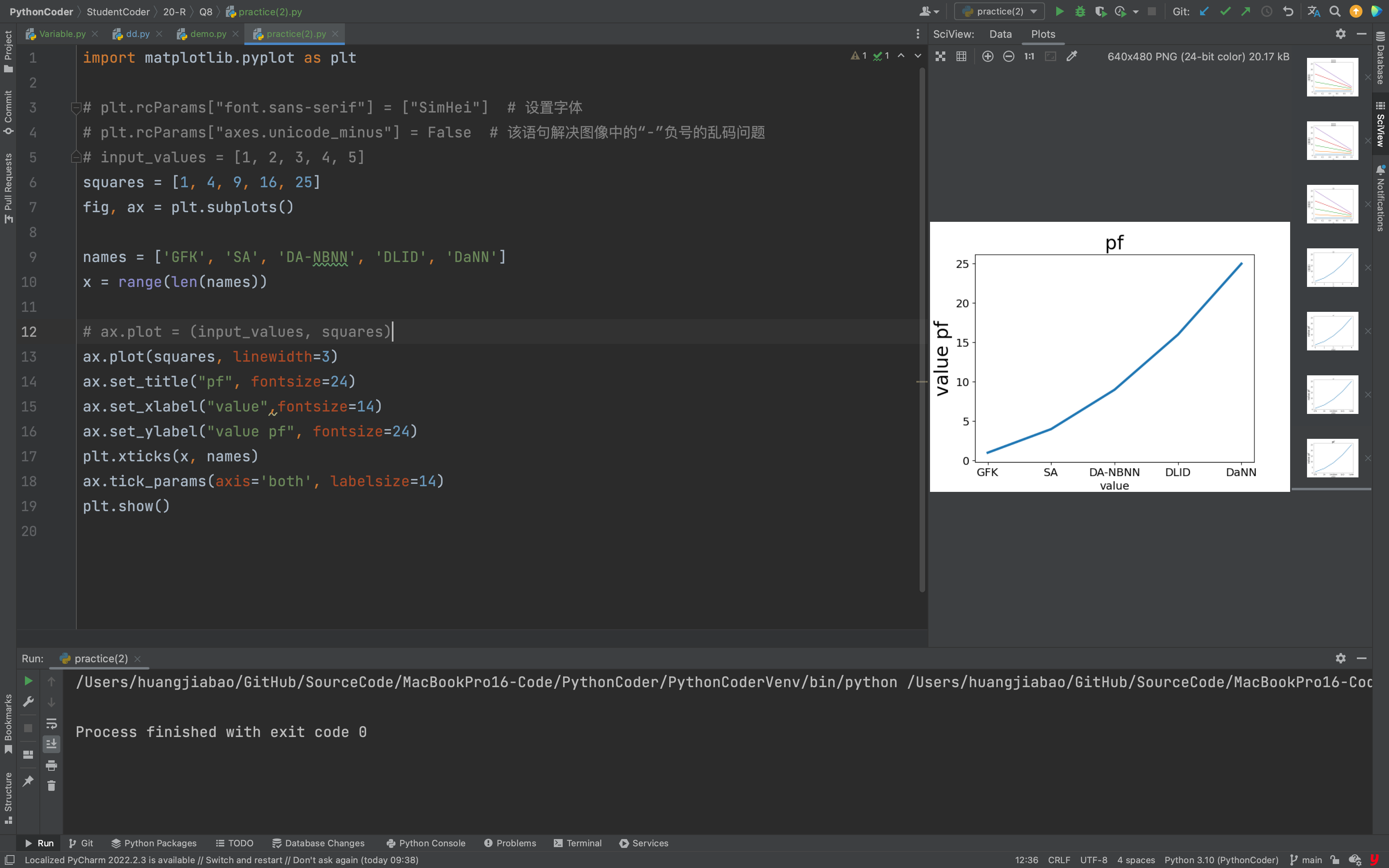
## Question 9
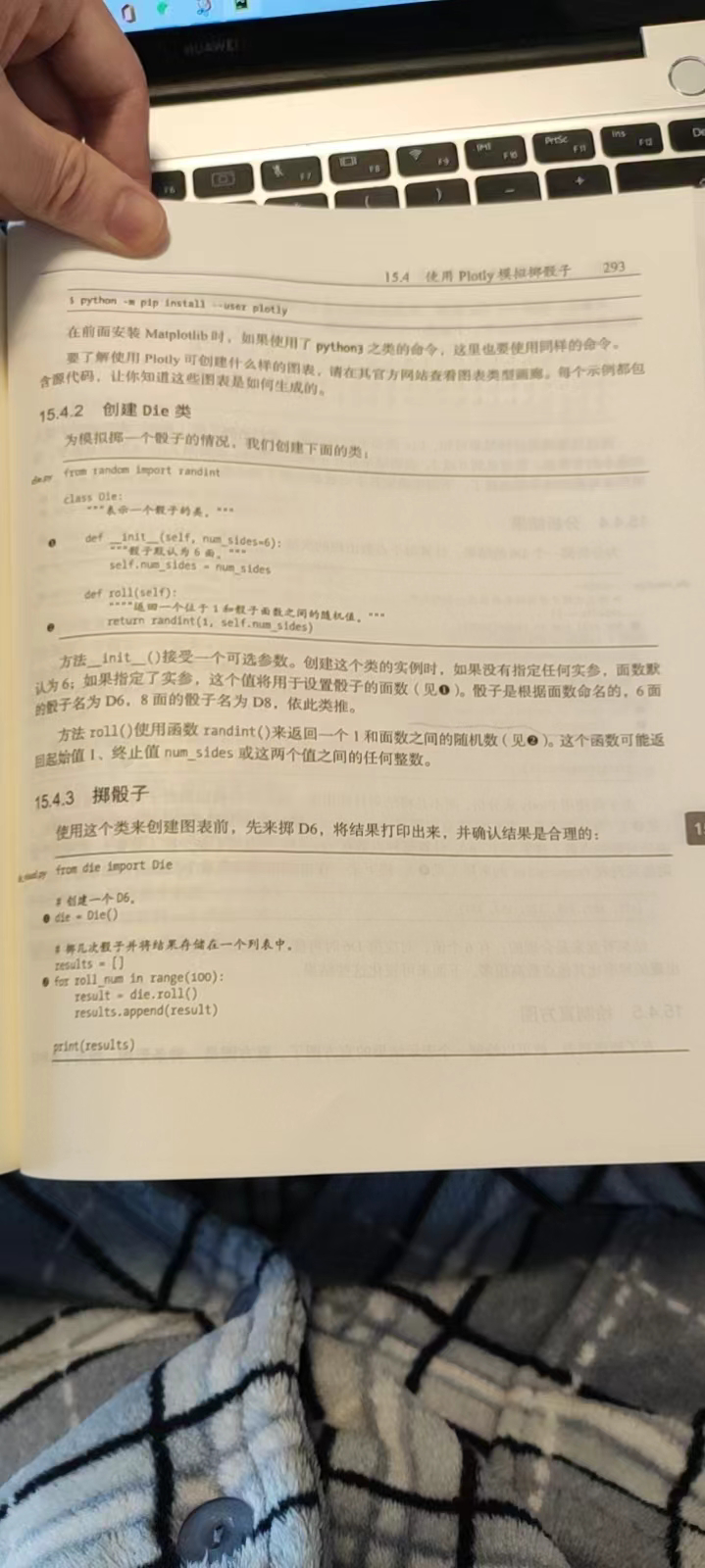
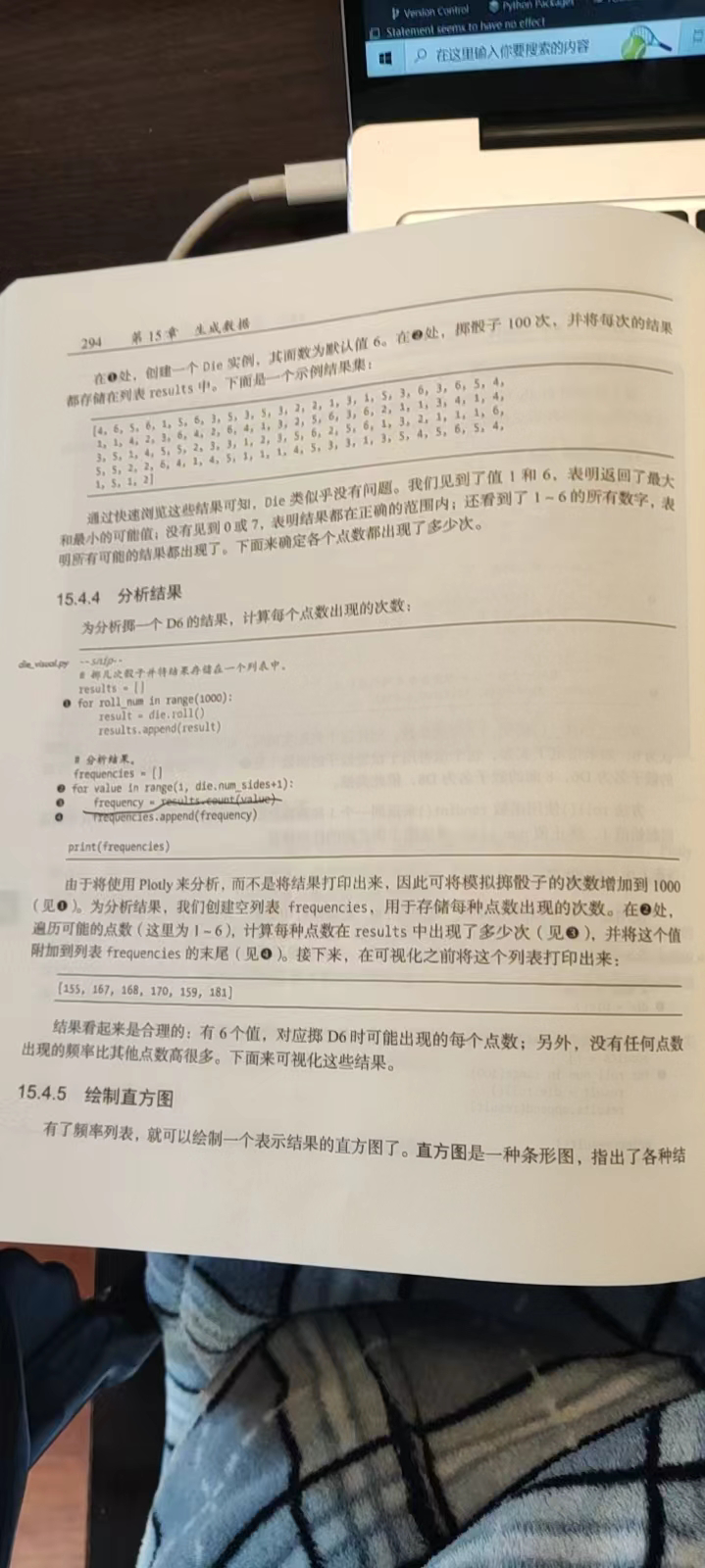
随机扔色子,用图表绘制出每一个数字被扔到的次数
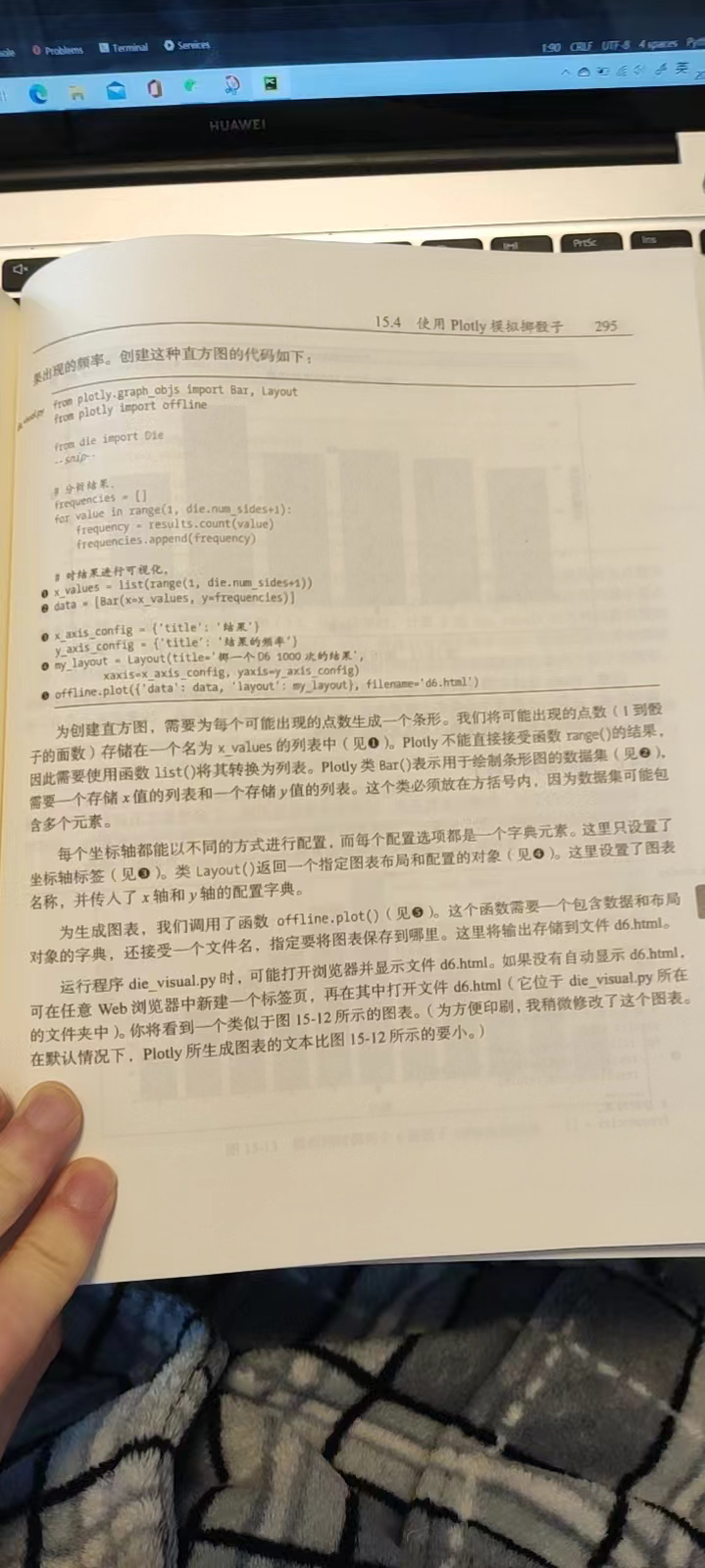
应该其他没有问题,就是最后一句吧我感觉


C:\Users\jin\Desktop\coder\venv\Scripts\python.exe C:\Users\jin\Desktop\coder\practice.py
Traceback (most recent call last):
File "C:\Users\jin\Desktop\coder\practice.py", line 23, in <module>
offline.plot({'data':data,'layout':my_latout},filename='d6.html')
File "C:\Users\jin\Desktop\coder\venv\lib\site-packages\plotly\offline\offline.py", line 586, in plot
pio.write_html(
File "C:\Users\jin\Desktop\coder\venv\lib\site-packages\plotly\io\_html.py", line 536, in write_html
path.write_text(html_str)
File "C:\Users\jin\AppData\Local\Programs\Python\Python39\lib\pathlib.py", line 1286, in write_text
return f.write(data)
UnicodeEncodeError: 'gbk' codec can't encode character '\u25c4' in position 276398: illegal multibyte sequence
Process finished with exit code 1
from random import randint
from plotly.graph_objs import Bar, Layout
from plotly import offline
class Die:
def __init__(self, num_sides=6):
self.num_sides = num_sides
def roll(self):
return randint(1, self.num_sides)
die = Die()
results = []
for roll_num in range(1000):
result = die.roll()
results.append(result)
frequencies = []
for value in range(1, die.num_sides + 1):
frequencey = results.count(value)
frequencies.append(frequencey)
x_values = list(range(1, die.num_sides + 1))
data = [Bar(x=x_values, y=frequencies)]
x_axis_config = {'title': '结果'}
y_axis_config = {'title': '结果的频率'}
my_latout = Layout(title='扔1000次的结果', xaxis=x_axis_config, yaxis=y_axis_config)
offline.plot({'data': data, 'layout': my_latout}, filename='d6.html')
你文字,全部用中文即可。
公众号:AI悦创【二维码】
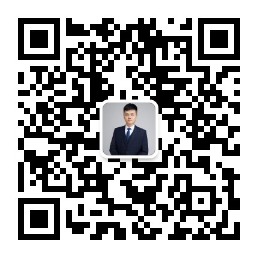
AI悦创·编程一对一
AI悦创·推出辅导班啦,包括「Python 语言辅导班、C++ 辅导班、java 辅导班、算法/数据结构辅导班、少儿编程、pygame 游戏开发、Web、Linux」,全部都是一对一教学:一对一辅导 + 一对一答疑 + 布置作业 + 项目实践等。当然,还有线下线上摄影课程、Photoshop、Premiere 一对一教学、QQ、微信在线,随时响应!微信:Jiabcdefh
C++ 信息奥赛题解,长期更新!长期招收一对一中小学信息奥赛集训,莆田、厦门地区有机会线下上门,其他地区线上。微信:Jiabcdefh
方法一:QQ
方法二:微信:Jiabcdefh
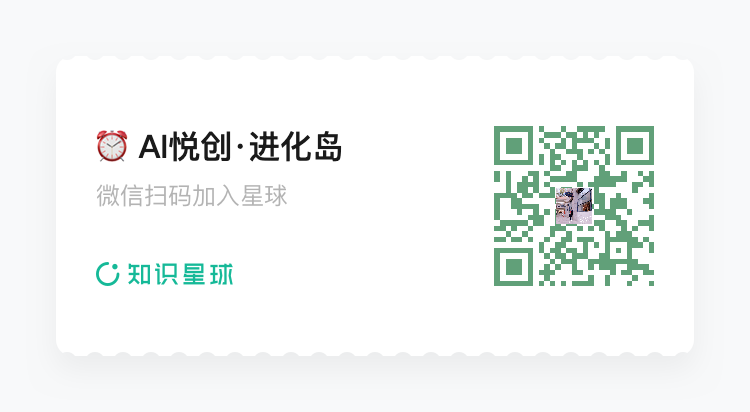
- 0
- 0
- 0
- 0
- 0
- 0